class: center, middle, inverse, title-slide .title[ # Stat 585 - APIs ] .author[ ### Heike Hofmann and Susan Vanderplas ] --- ## Application Programming Interfaces (APIs) - A set of instructions and standards for accessing structured information on the web - A very convenient way to get data from the internet - Usually, takes the form: `http://url.com?param1=value¶m2=value2` - Intended to make data available for other applications (e.g. developers of android apps, etc.) - More convenient than scraping HTML directly - Data is usually cleaner and better structured --- ## Reading API Documentation Most APIs come with some documentation: - [Petfinder API](https://www.petfinder.com/developers/api-docs) - [NOAA API](https://www.ncdc.noaa.gov/cdo-web/webservices/v2) - [List of data.gov APIs](https://catalog.data.gov/dataset?q=-aapi+api+OR++res_format%3Aapi#topic=developers_navigation) The documentation will tell you about - required authentication (and how to get an API key) - rate limits (how many requests you can make at a time) - query parameters and values --- ## API Authentication Many APIs will allow you to register and obtain an API key 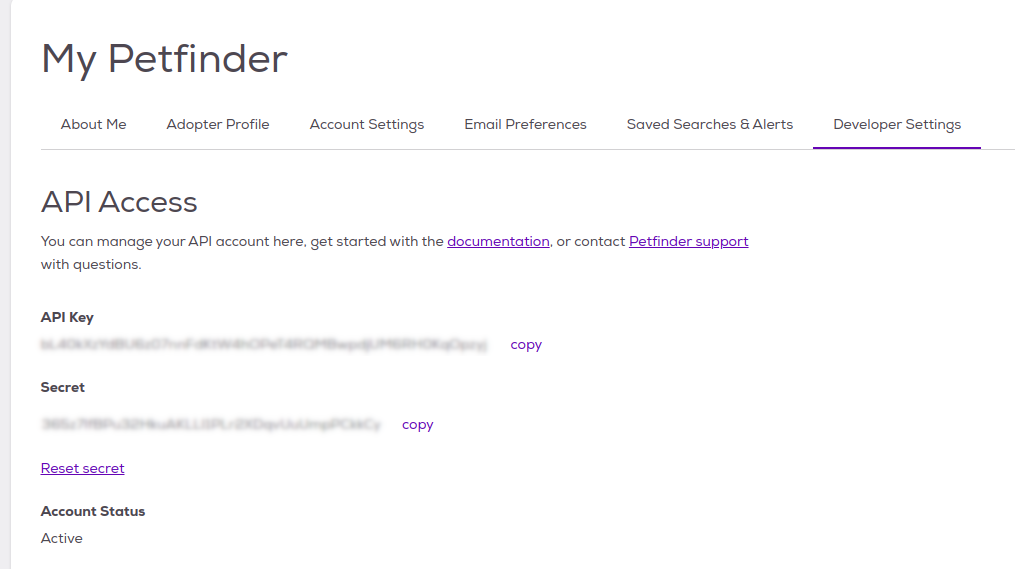 --- ## API Rate Limits APIs are a service provided for developers. Servers dedicated to these APIs are resource-limited, so be respectful of the data provider and the resource limits. 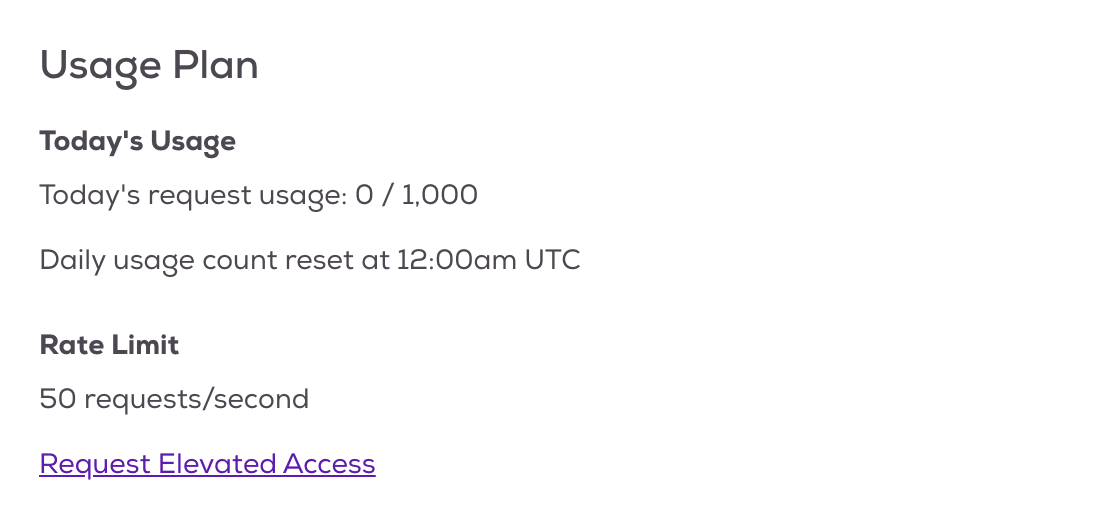 --- ## Getting Data 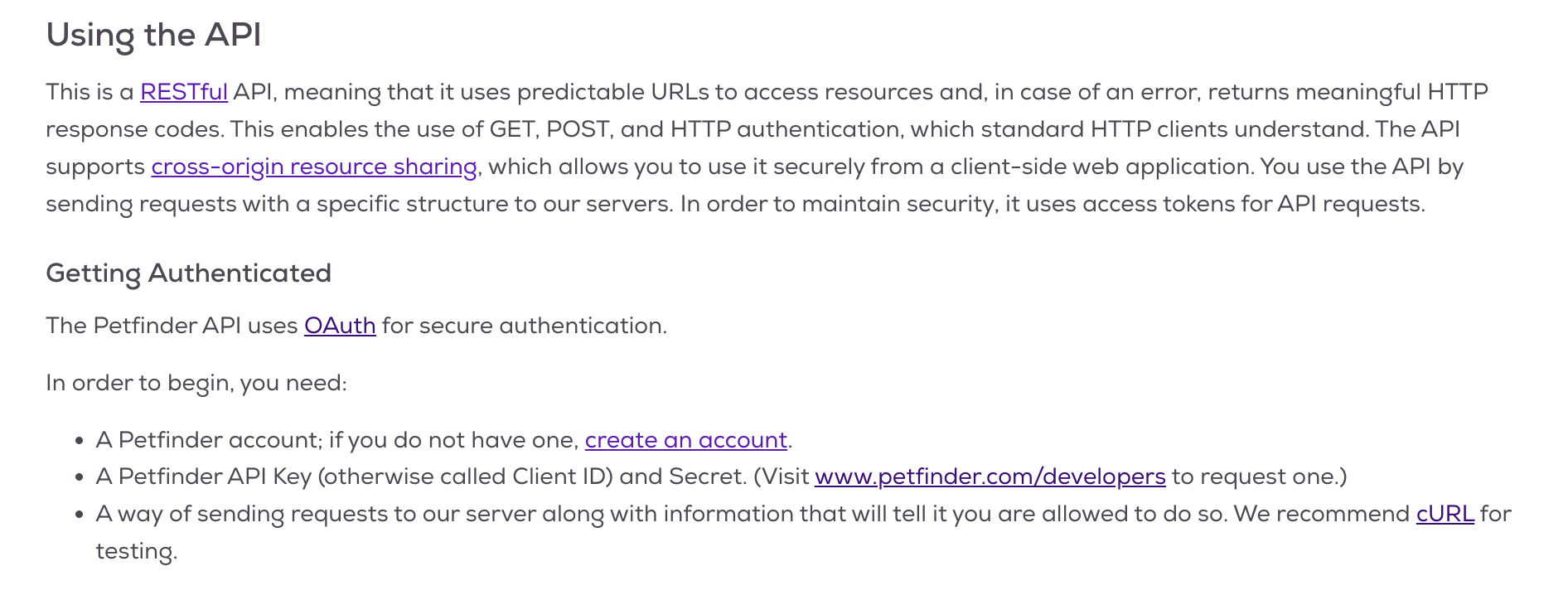 We will need to (1) Get our authorization token, then (2) build a request url: specify a category, then decide on an action, and any method search parameters --- ## Package `httr` allows us to work with the http protocol and map queries: ```r httr::POST( url = NULL, config = list(), ..., body = NULL, encode = c("multipart", "form", "json", "raw"), handle = NULL ) ``` POST allows us to let the website know about our - **identity**: through the key and the secret - **preferences**: which encoding do we want for the results? Result of a successful POST will contain our access token --- ## Getting the authorization token From the Petfinder website on getting an access token: ```r curl -d "grant_type=client_credentials&client_id={CLIENT-ID}& client_secret={CLIENT-SECRET}" https://api.petfinder.com/v2/oauth2/token ``` ```r library(rvest) library(xml2) key <- # Your key here secret <- # Your secret here endpoint <- 'oauth2/token' url <- "https://api.petfinder.com/v2/" req <- httr::POST(paste0(url, endpoint), body = list("grant_type"="client_credentials", "client_id" = key, "client_secret" = secret), encode="json" ) ``` Save the token: ```r token <- httr::content(req)$access_token ``` --- ## Categories and methods ```r curl -H "Authorization: Bearer {YOUR_ACCESS_TOKEN}" GET https://api.petfinder.com/v2/{CATEGORY}/ {ACTION}?{parameter_1}={value_1}&{parameter_2}={value_2} ``` Read through [categories](https://www.petfinder.com/developers/v2/docs/#get-animals), most general category: `animals` 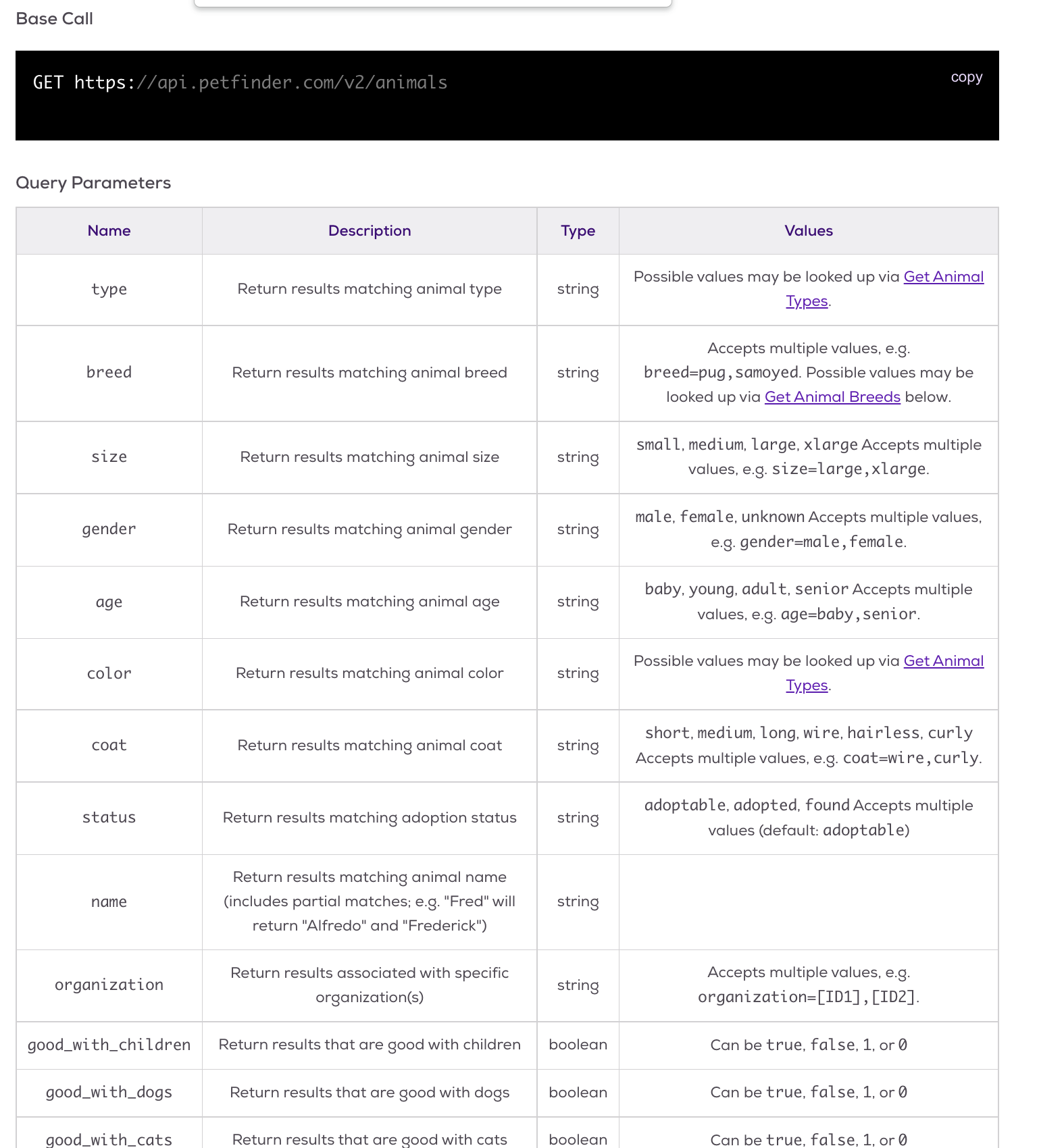 --- class: inverse ## Your Turn Assume, we want to find all of the cats that are good with children closest to Ames (zip code 50010) Which search parameters do we need to specify? What does the url look like? --- ## Your Turn - Solution cats are a *type*: type=cat good_with_children=true location=50010 The search string is therefore: ```r animals?type=cat&good_with_children=true&location=50010 ``` ``` ## [1] "Emmett Treasure Sopapilla" ``` 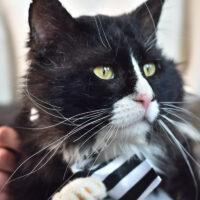<!-- -->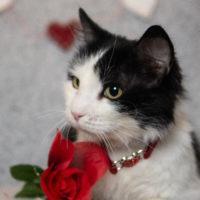<!-- -->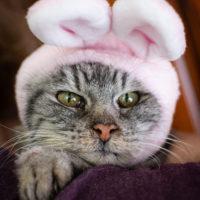<!-- --> --- ## Method `GET` from package `httr` ```r httr::GET(url = NULL, config = list(), ..., handle = NULL) ``` `url` is the url of the page we want `config` helps with additional setting - e.g `add_headers` for authorization --- ## Now get some actual data ```r # Now get actual data req_data <- httr::GET( paste0(url, "animals?type=dog&location=50010"), httr::add_headers(Authorization = paste0('Bearer ', token, sep = '')) ) ``` ```r ames_dogs <- httr::content(req_data, as = "parsed") str(ames_dogs) ``` ``` ## List of 2 ## $ animals :List of 20 ## ..$ :List of 26 ## .. ..$ id : int 62134825 ## .. ..$ organization_id : chr "IA264" ## .. ..$ url : chr "https://www.petfinder.com/dog/bo-62134825/ia/humboldt/humboldt-county-animal-shelter-ia264/?referrer_id=4ce2071"| __truncated__ ## .. ..$ type : chr "Dog" ## .. ..$ species : chr "Dog" ## .. ..$ breeds :List of 4 ## .. .. ..$ primary : chr "Australian Shepherd" ## .. .. ..$ secondary: chr "Collie" ## .. .. ..$ mixed : logi TRUE ## .. .. ..$ unknown : logi FALSE ## .. ..$ colors :List of 3 ## .. .. ..$ primary : chr "Tricolor (Brown, Black, & White)" ## .. .. ..$ secondary: NULL ## .. .. ..$ tertiary : NULL ## .. ..$ age : chr "Adult" ## .. ..$ gender : chr "Male" ## .. ..$ size : chr "Medium" ## .. ..$ coat : chr "Long" ## .. ..$ attributes :List of 5 ## .. .. ..$ spayed_neutered: logi TRUE ## .. .. ..$ house_trained : logi TRUE ## .. .. ..$ declawed : NULL ## .. .. ..$ special_needs : logi FALSE ## .. .. ..$ shots_current : logi TRUE ## .. ..$ environment :List of 3 ## .. .. ..$ children: logi TRUE ## .. .. ..$ dogs : logi TRUE ## .. .. ..$ cats : logi TRUE ## .. ..$ tags :List of 9 ## .. .. ..$ : chr "Friendly" ## .. .. ..$ : chr "Affectionate" ## .. .. ..$ : chr "Smart" ## .. .. ..$ : chr "Playful" ## .. .. ..$ : chr "Curious" ## .. .. ..$ : chr "Brave" ## .. .. ..$ : chr "Funny" ## .. .. ..$ : chr "Athletic" ## .. .. ..$ : chr "Loves kisses" ## .. ..$ name : chr "Bo" ## .. ..$ description : chr "This is one smart dude! He looks at you and is absolutely ready to learn and please you. Not only..." ## .. ..$ organization_animal_id: NULL ## .. ..$ photos :List of 1 ## .. .. ..$ :List of 4 ## .. .. .. ..$ small : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62134825/1/?bust=1680632275&width=100" ## .. .. .. ..$ medium: chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62134825/1/?bust=1680632275&width=300" ## .. .. .. ..$ large : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62134825/1/?bust=1680632275&width=600" ## .. .. .. ..$ full : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62134825/1/?bust=1680632275" ## .. ..$ primary_photo_cropped :List of 4 ## .. .. ..$ small : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62134825/1/?bust=1680632275&width=300" ## .. .. ..$ medium: chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62134825/1/?bust=1680632275&width=450" ## .. .. ..$ large : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62134825/1/?bust=1680632275&width=600" ## .. .. ..$ full : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62134825/1/?bust=1680632275" ## .. ..$ videos : list() ## .. ..$ status : chr "adoptable" ## .. ..$ status_changed_at : chr "2023-04-04T18:17:58+0000" ## .. ..$ published_at : chr "2023-04-04T18:17:56+0000" ## .. ..$ distance : num 56.7 ## .. ..$ contact :List of 3 ## .. .. ..$ email : chr "Moffitt.adopt@gmail.com" ## .. .. ..$ phone : chr "(515) 604-6242" ## .. .. ..$ address:List of 6 ## .. .. .. ..$ address1: NULL ## .. .. .. ..$ address2: NULL ## .. .. .. ..$ city : chr "Humboldt" ## .. .. .. ..$ state : chr "IA" ## .. .. .. ..$ postcode: chr "50548" ## .. .. .. ..$ country : chr "US" ## .. ..$ _links :List of 3 ## .. .. ..$ self :List of 1 ## .. .. .. ..$ href: chr "/v2/animals/62134825" ## .. .. ..$ type :List of 1 ## .. .. .. ..$ href: chr "/v2/types/dog" ## .. .. ..$ organization:List of 1 ## .. .. .. ..$ href: chr "/v2/organizations/ia264" ## ..$ :List of 26 ## .. ..$ id : int 62133467 ## .. ..$ organization_id : chr "IA25" ## .. ..$ url : chr "https://www.petfinder.com/dog/minerva-62133467/ia/oskaloosa/stephen-memorial-animal-shelter-ia25/?referrer_id=4"| __truncated__ ## .. ..$ type : chr "Dog" ## .. ..$ species : chr "Dog" ## .. ..$ breeds :List of 4 ## .. .. ..$ primary : chr "German Shepherd Dog" ## .. .. ..$ secondary: NULL ## .. .. ..$ mixed : logi TRUE ## .. .. ..$ unknown : logi FALSE ## .. ..$ colors :List of 3 ## .. .. ..$ primary : NULL ## .. .. ..$ secondary: NULL ## .. .. ..$ tertiary : NULL ## .. ..$ age : chr "Young" ## .. ..$ gender : chr "Female" ## .. ..$ size : chr "Medium" ## .. ..$ coat : NULL ## .. ..$ attributes :List of 5 ## .. .. ..$ spayed_neutered: logi FALSE ## .. .. ..$ house_trained : logi FALSE ## .. .. ..$ declawed : NULL ## .. .. ..$ special_needs : logi FALSE ## .. .. ..$ shots_current : logi FALSE ## .. ..$ environment :List of 3 ## .. .. ..$ children: logi TRUE ## .. .. ..$ dogs : logi TRUE ## .. .. ..$ cats : NULL ## .. ..$ tags : list() ## .. ..$ name : chr "Minerva" ## .. ..$ description : NULL ## .. ..$ organization_animal_id: chr "19115832" ## .. ..$ photos : list() ## .. ..$ primary_photo_cropped : NULL ## .. ..$ videos : list() ## .. ..$ status : chr "adoptable" ## .. ..$ status_changed_at : chr "2023-04-04T17:30:48+0000" ## .. ..$ published_at : chr "2023-04-04T17:30:47+0000" ## .. ..$ distance : num 70.1 ## .. ..$ contact :List of 3 ## .. .. ..$ email : chr "smasadoptions@mahaska.org" ## .. .. ..$ phone : chr "(641) 673-3991" ## .. .. ..$ address:List of 6 ## .. .. .. ..$ address1: chr "1716 Pella Ave" ## .. .. .. ..$ address2: NULL ## .. .. .. ..$ city : chr "Oskaloosa" ## .. .. .. ..$ state : chr "IA" ## .. .. .. ..$ postcode: chr "52577" ## .. .. .. ..$ country : chr "US" ## .. ..$ _links :List of 3 ## .. .. ..$ self :List of 1 ## .. .. .. ..$ href: chr "/v2/animals/62133467" ## .. .. ..$ type :List of 1 ## .. .. .. ..$ href: chr "/v2/types/dog" ## .. .. ..$ organization:List of 1 ## .. .. .. ..$ href: chr "/v2/organizations/ia25" ## ..$ :List of 26 ## .. ..$ id : int 62133001 ## .. ..$ organization_id : chr "IA14" ## .. ..$ url : chr "https://www.petfinder.com/dog/tucker-62133001/ia/des-moines/animal-rescue-league-of-iowa-inc-ia14/?referrer_id="| __truncated__ ## .. ..$ type : chr "Dog" ## .. ..$ species : chr "Dog" ## .. ..$ breeds :List of 4 ## .. .. ..$ primary : chr "Shepherd" ## .. .. ..$ secondary: NULL ## .. .. ..$ mixed : logi TRUE ## .. .. ..$ unknown : logi FALSE ## .. ..$ colors :List of 3 ## .. .. ..$ primary : NULL ## .. .. ..$ secondary: NULL ## .. .. ..$ tertiary : NULL ## .. ..$ age : chr "Young" ## .. ..$ gender : chr "Male" ## .. ..$ size : chr "Medium" ## .. ..$ coat : NULL ## .. ..$ attributes :List of 5 ## .. .. ..$ spayed_neutered: logi TRUE ## .. .. ..$ house_trained : logi FALSE ## .. .. ..$ declawed : NULL ## .. .. ..$ special_needs : logi FALSE ## .. .. ..$ shots_current : logi TRUE ## .. ..$ environment :List of 3 ## .. .. ..$ children: NULL ## .. .. ..$ dogs : NULL ## .. .. ..$ cats : NULL ## .. ..$ tags : list() ## .. ..$ name : chr "Tucker" ## .. ..$ description : chr "Primary Color: Black Secondary Color: Tan Weight: 24.7lbs Age: 0yrs 6mths 3wks Animal has been Neutered" ## .. ..$ organization_animal_id: chr "229419" ## .. ..$ photos : list() ## .. ..$ primary_photo_cropped : NULL ## .. ..$ videos : list() ## .. ..$ status : chr "adoptable" ## .. ..$ status_changed_at : chr "2023-04-04T17:02:43+0000" ## .. ..$ published_at : chr "2023-04-04T17:02:42+0000" ## .. ..$ distance : num 26.2 ## .. ..$ contact :List of 3 ## .. .. ..$ email : chr "social@arl-iowa.org" ## .. .. ..$ phone : NULL ## .. .. ..$ address:List of 6 ## .. .. .. ..$ address1: chr "5452 N.E. 22nd St." ## .. .. .. ..$ address2: NULL ## .. .. .. ..$ city : chr "Des Moines" ## .. .. .. ..$ state : chr "IA" ## .. .. .. ..$ postcode: chr "50313" ## .. .. .. ..$ country : chr "US" ## .. ..$ _links :List of 3 ## .. .. ..$ self :List of 1 ## .. .. .. ..$ href: chr "/v2/animals/62133001" ## .. .. ..$ type :List of 1 ## .. .. .. ..$ href: chr "/v2/types/dog" ## .. .. ..$ organization:List of 1 ## .. .. .. ..$ href: chr "/v2/organizations/ia14" ## ..$ :List of 26 ## .. ..$ id : int 62133002 ## .. ..$ organization_id : chr "IA14" ## .. ..$ url : chr "https://www.petfinder.com/dog/pubby-62133002/ia/des-moines/animal-rescue-league-of-iowa-inc-ia14/?referrer_id=4"| __truncated__ ## .. ..$ type : chr "Dog" ## .. ..$ species : chr "Dog" ## .. ..$ breeds :List of 4 ## .. .. ..$ primary : chr "Pit Bull Terrier" ## .. .. ..$ secondary: NULL ## .. .. ..$ mixed : logi TRUE ## .. .. ..$ unknown : logi FALSE ## .. ..$ colors :List of 3 ## .. .. ..$ primary : NULL ## .. .. ..$ secondary: NULL ## .. .. ..$ tertiary : NULL ## .. ..$ age : chr "Young" ## .. ..$ gender : chr "Male" ## .. ..$ size : chr "Medium" ## .. ..$ coat : NULL ## .. ..$ attributes :List of 5 ## .. .. ..$ spayed_neutered: logi TRUE ## .. .. ..$ house_trained : logi FALSE ## .. .. ..$ declawed : NULL ## .. .. ..$ special_needs : logi FALSE ## .. .. ..$ shots_current : logi TRUE ## .. ..$ environment :List of 3 ## .. .. ..$ children: NULL ## .. .. ..$ dogs : NULL ## .. .. ..$ cats : NULL ## .. ..$ tags : list() ## .. ..$ name : chr "Pubby" ## .. ..$ description : chr "Primary Color: White Secondary Color: Grey Weight: 45lbs Age: 0yrs 11mths 3wks Animal has been Neutered" ## .. ..$ organization_animal_id: chr "229406" ## .. ..$ photos : list() ## .. ..$ primary_photo_cropped : NULL ## .. ..$ videos : list() ## .. ..$ status : chr "adoptable" ## .. ..$ status_changed_at : chr "2023-04-04T17:02:43+0000" ## .. ..$ published_at : chr "2023-04-04T17:02:42+0000" ## .. ..$ distance : num 26.2 ## .. ..$ contact :List of 3 ## .. .. ..$ email : chr "social@arl-iowa.org" ## .. .. ..$ phone : NULL ## .. .. ..$ address:List of 6 ## .. .. .. ..$ address1: chr "5452 N.E. 22nd St." ## .. .. .. ..$ address2: NULL ## .. .. .. ..$ city : chr "Des Moines" ## .. .. .. ..$ state : chr "IA" ## .. .. .. ..$ postcode: chr "50313" ## .. .. .. ..$ country : chr "US" ## .. ..$ _links :List of 3 ## .. .. ..$ self :List of 1 ## .. .. .. ..$ href: chr "/v2/animals/62133002" ## .. .. ..$ type :List of 1 ## .. .. .. ..$ href: chr "/v2/types/dog" ## .. .. ..$ organization:List of 1 ## .. .. .. ..$ href: chr "/v2/organizations/ia14" ## ..$ :List of 26 ## .. ..$ id : int 62133000 ## .. ..$ organization_id : chr "IA14" ## .. ..$ url : chr "https://www.petfinder.com/dog/diesel-62133000/ia/des-moines/animal-rescue-league-of-iowa-inc-ia14/?referrer_id="| __truncated__ ## .. ..$ type : chr "Dog" ## .. ..$ species : chr "Dog" ## .. ..$ breeds :List of 4 ## .. .. ..$ primary : chr "Rottweiler" ## .. .. ..$ secondary: NULL ## .. .. ..$ mixed : logi TRUE ## .. .. ..$ unknown : logi FALSE ## .. ..$ colors :List of 3 ## .. .. ..$ primary : NULL ## .. .. ..$ secondary: NULL ## .. .. ..$ tertiary : NULL ## .. ..$ age : chr "Baby" ## .. ..$ gender : chr "Male" ## .. ..$ size : chr "Small" ## .. ..$ coat : NULL ## .. ..$ attributes :List of 5 ## .. .. ..$ spayed_neutered: logi TRUE ## .. .. ..$ house_trained : logi FALSE ## .. .. ..$ declawed : NULL ## .. .. ..$ special_needs : logi FALSE ## .. .. ..$ shots_current : logi TRUE ## .. ..$ environment :List of 3 ## .. .. ..$ children: NULL ## .. .. ..$ dogs : NULL ## .. .. ..$ cats : NULL ## .. ..$ tags : list() ## .. ..$ name : chr "Diesel" ## .. ..$ description : chr "Primary Color: Black Secondary Color: Tan Weight: 20.88lbs Age: 0yrs 0mths 11wks Animal has been Neutered" ## .. ..$ organization_animal_id: chr "229941" ## .. ..$ photos : list() ## .. ..$ primary_photo_cropped : NULL ## .. ..$ videos : list() ## .. ..$ status : chr "adoptable" ## .. ..$ status_changed_at : chr "2023-04-04T17:02:44+0000" ## .. ..$ published_at : chr "2023-04-04T17:02:42+0000" ## .. ..$ distance : num 26.2 ## .. ..$ contact :List of 3 ## .. .. ..$ email : chr "social@arl-iowa.org" ## .. .. ..$ phone : NULL ## .. .. ..$ address:List of 6 ## .. .. .. ..$ address1: chr "5452 N.E. 22nd St." ## .. .. .. ..$ address2: NULL ## .. .. .. ..$ city : chr "Des Moines" ## .. .. .. ..$ state : chr "IA" ## .. .. .. ..$ postcode: chr "50313" ## .. .. .. ..$ country : chr "US" ## .. ..$ _links :List of 3 ## .. .. ..$ self :List of 1 ## .. .. .. ..$ href: chr "/v2/animals/62133000" ## .. .. ..$ type :List of 1 ## .. .. .. ..$ href: chr "/v2/types/dog" ## .. .. ..$ organization:List of 1 ## .. .. .. ..$ href: chr "/v2/organizations/ia14" ## ..$ :List of 26 ## .. ..$ id : int 62133004 ## .. ..$ organization_id : chr "IA14" ## .. ..$ url : chr "https://www.petfinder.com/dog/ghost-62133004/ia/des-moines/animal-rescue-league-of-iowa-inc-ia14/?referrer_id=4"| __truncated__ ## .. ..$ type : chr "Dog" ## .. ..$ species : chr "Dog" ## .. ..$ breeds :List of 4 ## .. .. ..$ primary : chr "Labrador Retriever" ## .. .. ..$ secondary: NULL ## .. .. ..$ mixed : logi TRUE ## .. .. ..$ unknown : logi FALSE ## .. ..$ colors :List of 3 ## .. .. ..$ primary : NULL ## .. .. ..$ secondary: NULL ## .. .. ..$ tertiary : NULL ## .. ..$ age : chr "Young" ## .. ..$ gender : chr "Male" ## .. ..$ size : chr "Large" ## .. ..$ coat : NULL ## .. ..$ attributes :List of 5 ## .. .. ..$ spayed_neutered: logi TRUE ## .. .. ..$ house_trained : logi FALSE ## .. .. ..$ declawed : NULL ## .. .. ..$ special_needs : logi FALSE ## .. .. ..$ shots_current : logi TRUE ## .. ..$ environment :List of 3 ## .. .. ..$ children: NULL ## .. .. ..$ dogs : NULL ## .. .. ..$ cats : NULL ## .. ..$ tags : list() ## .. ..$ name : chr "Ghost" ## .. ..$ description : chr "Primary Color: Merle Secondary Color: White Weight: 48.2lbs Age: 0yrs 11mths 1wks Animal has been Neutered" ## .. ..$ organization_animal_id: chr "229533" ## .. ..$ photos :List of 3 ## .. .. ..$ :List of 4 ## .. .. .. ..$ small : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62133004/1/?bust=1680633569&width=100" ## .. .. .. ..$ medium: chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62133004/1/?bust=1680633569&width=300" ## .. .. .. ..$ large : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62133004/1/?bust=1680633569&width=600" ## .. .. .. ..$ full : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62133004/1/?bust=1680633569" ## .. .. ..$ :List of 4 ## .. .. .. ..$ small : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62133004/2/?bust=1680632946&width=100" ## .. .. .. ..$ medium: chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62133004/2/?bust=1680632946&width=300" ## .. .. .. ..$ large : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62133004/2/?bust=1680632946&width=600" ## .. .. .. ..$ full : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62133004/2/?bust=1680632946" ## .. .. ..$ :List of 4 ## .. .. .. ..$ small : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62133004/3/?bust=1680633101&width=100" ## .. .. .. ..$ medium: chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62133004/3/?bust=1680633101&width=300" ## .. .. .. ..$ large : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62133004/3/?bust=1680633101&width=600" ## .. .. .. ..$ full : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62133004/3/?bust=1680633101" ## .. ..$ primary_photo_cropped :List of 4 ## .. .. ..$ small : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62133004/1/?bust=1680633569&width=300" ## .. .. ..$ medium: chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62133004/1/?bust=1680633569&width=450" ## .. .. ..$ large : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62133004/1/?bust=1680633569&width=600" ## .. .. ..$ full : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62133004/1/?bust=1680633569" ## .. ..$ videos : list() ## .. ..$ status : chr "adoptable" ## .. ..$ status_changed_at : chr "2023-04-04T17:02:44+0000" ## .. ..$ published_at : chr "2023-04-04T17:02:42+0000" ## .. ..$ distance : num 26.2 ## .. ..$ contact :List of 3 ## .. .. ..$ email : chr "social@arl-iowa.org" ## .. .. ..$ phone : NULL ## .. .. ..$ address:List of 6 ## .. .. .. ..$ address1: chr "5452 N.E. 22nd St." ## .. .. .. ..$ address2: NULL ## .. .. .. ..$ city : chr "Des Moines" ## .. .. .. ..$ state : chr "IA" ## .. .. .. ..$ postcode: chr "50313" ## .. .. .. ..$ country : chr "US" ## .. ..$ _links :List of 3 ## .. .. ..$ self :List of 1 ## .. .. .. ..$ href: chr "/v2/animals/62133004" ## .. .. ..$ type :List of 1 ## .. .. .. ..$ href: chr "/v2/types/dog" ## .. .. ..$ organization:List of 1 ## .. .. .. ..$ href: chr "/v2/organizations/ia14" ## ..$ :List of 26 ## .. ..$ id : int 62132677 ## .. ..$ organization_id : chr "IA14" ## .. ..$ url : chr "https://www.petfinder.com/dog/frankie-62132677/ia/des-moines/animal-rescue-league-of-iowa-inc-ia14/?referrer_id"| __truncated__ ## .. ..$ type : chr "Dog" ## .. ..$ species : chr "Dog" ## .. ..$ breeds :List of 4 ## .. .. ..$ primary : chr "German Shepherd Dog" ## .. .. ..$ secondary: NULL ## .. .. ..$ mixed : logi TRUE ## .. .. ..$ unknown : logi FALSE ## .. ..$ colors :List of 3 ## .. .. ..$ primary : NULL ## .. .. ..$ secondary: NULL ## .. .. ..$ tertiary : NULL ## .. ..$ age : chr "Young" ## .. ..$ gender : chr "Male" ## .. ..$ size : chr "Large" ## .. ..$ coat : NULL ## .. ..$ attributes :List of 5 ## .. .. ..$ spayed_neutered: logi TRUE ## .. .. ..$ house_trained : logi FALSE ## .. .. ..$ declawed : NULL ## .. .. ..$ special_needs : logi FALSE ## .. .. ..$ shots_current : logi TRUE ## .. ..$ environment :List of 3 ## .. .. ..$ children: NULL ## .. .. ..$ dogs : NULL ## .. .. ..$ cats : NULL ## .. ..$ tags : list() ## .. ..$ name : chr "Frankie" ## .. ..$ description : chr "Primary Color: Tan Secondary Color: Black Age: 0yrs 8mths 2wks Animal has been Neutered" ## .. ..$ organization_animal_id: chr "229833" ## .. ..$ photos : list() ## .. ..$ primary_photo_cropped : NULL ## .. ..$ videos : list() ## .. ..$ status : chr "adoptable" ## .. ..$ status_changed_at : chr "2023-04-04T16:47:33+0000" ## .. ..$ published_at : chr "2023-04-04T16:47:32+0000" ## .. ..$ distance : num 26.2 ## .. ..$ contact :List of 3 ## .. .. ..$ email : chr "social@arl-iowa.org" ## .. .. ..$ phone : NULL ## .. .. ..$ address:List of 6 ## .. .. .. ..$ address1: chr "5452 N.E. 22nd St." ## .. .. .. ..$ address2: NULL ## .. .. .. ..$ city : chr "Des Moines" ## .. .. .. ..$ state : chr "IA" ## .. .. .. ..$ postcode: chr "50313" ## .. .. .. ..$ country : chr "US" ## .. ..$ _links :List of 3 ## .. .. ..$ self :List of 1 ## .. .. .. ..$ href: chr "/v2/animals/62132677" ## .. .. ..$ type :List of 1 ## .. .. .. ..$ href: chr "/v2/types/dog" ## .. .. ..$ organization:List of 1 ## .. .. .. ..$ href: chr "/v2/organizations/ia14" ## ..$ :List of 26 ## .. ..$ id : int 62132676 ## .. ..$ organization_id : chr "IA14" ## .. ..$ url : chr "https://www.petfinder.com/dog/gambler-62132676/ia/des-moines/animal-rescue-league-of-iowa-inc-ia14/?referrer_id"| __truncated__ ## .. ..$ type : chr "Dog" ## .. ..$ species : chr "Dog" ## .. ..$ breeds :List of 4 ## .. .. ..$ primary : chr "Australian Shepherd" ## .. .. ..$ secondary: NULL ## .. .. ..$ mixed : logi TRUE ## .. .. ..$ unknown : logi FALSE ## .. ..$ colors :List of 3 ## .. .. ..$ primary : NULL ## .. .. ..$ secondary: NULL ## .. .. ..$ tertiary : NULL ## .. ..$ age : chr "Adult" ## .. ..$ gender : chr "Male" ## .. ..$ size : chr "Medium" ## .. ..$ coat : NULL ## .. ..$ attributes :List of 5 ## .. .. ..$ spayed_neutered: logi TRUE ## .. .. ..$ house_trained : logi FALSE ## .. .. ..$ declawed : NULL ## .. .. ..$ special_needs : logi FALSE ## .. .. ..$ shots_current : logi TRUE ## .. ..$ environment :List of 3 ## .. .. ..$ children: NULL ## .. .. ..$ dogs : NULL ## .. .. ..$ cats : NULL ## .. ..$ tags : list() ## .. ..$ name : chr "Gambler" ## .. ..$ description : chr "Primary Color: Black Secondary Color: White Weight: 51lbs Age: 2yrs 0mths 0wks Animal has been Neutered" ## .. ..$ organization_animal_id: chr "229848" ## .. ..$ photos :List of 1 ## .. .. ..$ :List of 4 ## .. .. .. ..$ small : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62132676/1/?bust=1680632546&width=100" ## .. .. .. ..$ medium: chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62132676/1/?bust=1680632546&width=300" ## .. .. .. ..$ large : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62132676/1/?bust=1680632546&width=600" ## .. .. .. ..$ full : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62132676/1/?bust=1680632546" ## .. ..$ primary_photo_cropped :List of 4 ## .. .. ..$ small : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62132676/1/?bust=1680632546&width=300" ## .. .. ..$ medium: chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62132676/1/?bust=1680632546&width=450" ## .. .. ..$ large : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62132676/1/?bust=1680632546&width=600" ## .. .. ..$ full : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62132676/1/?bust=1680632546" ## .. ..$ videos : list() ## .. ..$ status : chr "adoptable" ## .. ..$ status_changed_at : chr "2023-04-04T16:47:35+0000" ## .. ..$ published_at : chr "2023-04-04T16:47:32+0000" ## .. ..$ distance : num 26.2 ## .. ..$ contact :List of 3 ## .. .. ..$ email : chr "social@arl-iowa.org" ## .. .. ..$ phone : NULL ## .. .. ..$ address:List of 6 ## .. .. .. ..$ address1: chr "5452 N.E. 22nd St." ## .. .. .. ..$ address2: NULL ## .. .. .. ..$ city : chr "Des Moines" ## .. .. .. ..$ state : chr "IA" ## .. .. .. ..$ postcode: chr "50313" ## .. .. .. ..$ country : chr "US" ## .. ..$ _links :List of 3 ## .. .. ..$ self :List of 1 ## .. .. .. ..$ href: chr "/v2/animals/62132676" ## .. .. ..$ type :List of 1 ## .. .. .. ..$ href: chr "/v2/types/dog" ## .. .. ..$ organization:List of 1 ## .. .. .. ..$ href: chr "/v2/organizations/ia14" ## ..$ :List of 26 ## .. ..$ id : int 62131790 ## .. ..$ organization_id : chr "IA36" ## .. ..$ url : chr "https://www.petfinder.com/dog/lucille-62131790/ia/waterloo/cedar-bend-humane-society-ia36/?referrer_id=4ce20718"| __truncated__ ## .. ..$ type : chr "Dog" ## .. ..$ species : chr "Dog" ## .. ..$ breeds :List of 4 ## .. .. ..$ primary : chr "Australian Shepherd" ## .. .. ..$ secondary: NULL ## .. .. ..$ mixed : logi FALSE ## .. .. ..$ unknown : logi FALSE ## .. ..$ colors :List of 3 ## .. .. ..$ primary : chr "Merle (Red)" ## .. .. ..$ secondary: chr "White / Cream" ## .. .. ..$ tertiary : NULL ## .. ..$ age : chr "Adult" ## .. ..$ gender : chr "Female" ## .. ..$ size : chr "Large" ## .. ..$ coat : chr "Medium" ## .. ..$ attributes :List of 5 ## .. .. ..$ spayed_neutered: logi FALSE ## .. .. ..$ house_trained : logi FALSE ## .. .. ..$ declawed : NULL ## .. .. ..$ special_needs : logi FALSE ## .. .. ..$ shots_current : logi TRUE ## .. ..$ environment :List of 3 ## .. .. ..$ children: NULL ## .. .. ..$ dogs : NULL ## .. .. ..$ cats : NULL ## .. ..$ tags :List of 9 ## .. .. ..$ : chr "Friendly" ## .. .. ..$ : chr "Affectionate" ## .. .. ..$ : chr "Curious" ## .. .. ..$ : chr "Athletic" ## .. .. ..$ : chr "Funny" ## .. .. ..$ : chr "Playful" ## .. .. ..$ : chr "Smart" ## .. .. ..$ : chr "Loves kisses" ## .. .. ..$ : chr "Timid" ## .. ..$ name : chr "Lucille " ## .. ..$ description : chr "MY STORY: Lucille came to CBHS as a stray.\n\nAGE: Approximately 3 years old. \n\nHi, I'm Lucille.\nNice to meet..." ## .. ..$ organization_animal_id: NULL ## .. ..$ photos :List of 3 ## .. .. ..$ :List of 4 ## .. .. .. ..$ small : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131790/1/?bust=1680623359&width=100" ## .. .. .. ..$ medium: chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131790/1/?bust=1680623359&width=300" ## .. .. .. ..$ large : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131790/1/?bust=1680623359&width=600" ## .. .. .. ..$ full : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131790/1/?bust=1680623359" ## .. .. ..$ :List of 4 ## .. .. .. ..$ small : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131790/2/?bust=1680624908&width=100" ## .. .. .. ..$ medium: chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131790/2/?bust=1680624908&width=300" ## .. .. .. ..$ large : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131790/2/?bust=1680624908&width=600" ## .. .. .. ..$ full : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131790/2/?bust=1680624908" ## .. .. ..$ :List of 4 ## .. .. .. ..$ small : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131790/3/?bust=1680623361&width=100" ## .. .. .. ..$ medium: chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131790/3/?bust=1680623361&width=300" ## .. .. .. ..$ large : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131790/3/?bust=1680623361&width=600" ## .. .. .. ..$ full : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131790/3/?bust=1680623361" ## .. ..$ primary_photo_cropped :List of 4 ## .. .. ..$ small : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131790/1/?bust=1680623359&width=300" ## .. .. ..$ medium: chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131790/1/?bust=1680623359&width=450" ## .. .. ..$ large : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131790/1/?bust=1680623359&width=600" ## .. .. ..$ full : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131790/1/?bust=1680623359" ## .. ..$ videos : list() ## .. ..$ status : chr "adoptable" ## .. ..$ status_changed_at : chr "2023-04-04T15:49:24+0000" ## .. ..$ published_at : chr "2023-04-04T15:49:22+0000" ## .. ..$ distance : num 72.3 ## .. ..$ contact :List of 3 ## .. .. ..$ email : chr "adoptioncounselor@cedarbendhumane.org" ## .. .. ..$ phone : chr "(319) 232-6887" ## .. .. ..$ address:List of 6 ## .. .. .. ..$ address1: chr "1166 West Airline Highway" ## .. .. .. ..$ address2: NULL ## .. .. .. ..$ city : chr "Waterloo" ## .. .. .. ..$ state : chr "IA" ## .. .. .. ..$ postcode: chr "50703" ## .. .. .. ..$ country : chr "US" ## .. ..$ _links :List of 3 ## .. .. ..$ self :List of 1 ## .. .. .. ..$ href: chr "/v2/animals/62131790" ## .. .. ..$ type :List of 1 ## .. .. .. ..$ href: chr "/v2/types/dog" ## .. .. ..$ organization:List of 1 ## .. .. .. ..$ href: chr "/v2/organizations/ia36" ## ..$ :List of 26 ## .. ..$ id : int 62131338 ## .. ..$ organization_id : chr "IA186" ## .. ..$ url : chr "https://www.petfinder.com/dog/dakota-62131338/ia/de-soto/aheinz57-pet-rescue-and-transport-inc-ia186/?referrer_"| __truncated__ ## .. ..$ type : chr "Dog" ## .. ..$ species : chr "Dog" ## .. ..$ breeds :List of 4 ## .. .. ..$ primary : chr "Cattle Dog" ## .. .. ..$ secondary: chr "Mixed Breed" ## .. .. ..$ mixed : logi TRUE ## .. .. ..$ unknown : logi FALSE ## .. ..$ colors :List of 3 ## .. .. ..$ primary : NULL ## .. .. ..$ secondary: NULL ## .. .. ..$ tertiary : NULL ## .. ..$ age : chr "Adult" ## .. ..$ gender : chr "Female" ## .. ..$ size : chr "Medium" ## .. ..$ coat : NULL ## .. ..$ attributes :List of 5 ## .. .. ..$ spayed_neutered: logi TRUE ## .. .. ..$ house_trained : logi FALSE ## .. .. ..$ declawed : NULL ## .. .. ..$ special_needs : logi FALSE ## .. .. ..$ shots_current : logi FALSE ## .. ..$ environment :List of 3 ## .. .. ..$ children: NULL ## .. .. ..$ dogs : NULL ## .. .. ..$ cats : NULL ## .. ..$ tags : list() ## .. ..$ name : chr "Dakota" ## .. ..$ description : NULL ## .. ..$ organization_animal_id: chr "52307794" ## .. ..$ photos :List of 2 ## .. .. ..$ :List of 4 ## .. .. .. ..$ small : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131338/1/?bust=1680624708&width=100" ## .. .. .. ..$ medium: chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131338/1/?bust=1680624708&width=300" ## .. .. .. ..$ large : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131338/1/?bust=1680624708&width=600" ## .. .. .. ..$ full : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131338/1/?bust=1680624708" ## .. .. ..$ :List of 4 ## .. .. .. ..$ small : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131338/2/?bust=1680624417&width=100" ## .. .. .. ..$ medium: chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131338/2/?bust=1680624417&width=300" ## .. .. .. ..$ large : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131338/2/?bust=1680624417&width=600" ## .. .. .. ..$ full : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131338/2/?bust=1680624417" ## .. ..$ primary_photo_cropped :List of 4 ## .. .. ..$ small : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131338/1/?bust=1680624708&width=300" ## .. .. ..$ medium: chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131338/1/?bust=1680624708&width=450" ## .. .. ..$ large : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131338/1/?bust=1680624708&width=600" ## .. .. ..$ full : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131338/1/?bust=1680624708" ## .. ..$ videos : list() ## .. ..$ status : chr "adoptable" ## .. ..$ status_changed_at : chr "2023-04-04T15:06:16+0000" ## .. ..$ published_at : chr "2023-04-04T15:06:14+0000" ## .. ..$ distance : num 40.4 ## .. ..$ contact :List of 3 ## .. .. ..$ email : chr "adopt57@aheinz57.com" ## .. .. ..$ phone : chr "(515) 834-1157" ## .. .. ..$ address:List of 6 ## .. .. .. ..$ address1: chr "4002 Ash Street" ## .. .. .. ..$ address2: NULL ## .. .. .. ..$ city : chr "De Soto" ## .. .. .. ..$ state : chr "IA" ## .. .. .. ..$ postcode: chr "50069" ## .. .. .. ..$ country : chr "US" ## .. ..$ _links :List of 3 ## .. .. ..$ self :List of 1 ## .. .. .. ..$ href: chr "/v2/animals/62131338" ## .. .. ..$ type :List of 1 ## .. .. .. ..$ href: chr "/v2/types/dog" ## .. .. ..$ organization:List of 1 ## .. .. .. ..$ href: chr "/v2/organizations/ia186" ## ..$ :List of 26 ## .. ..$ id : int 62131013 ## .. ..$ organization_id : chr "IA36" ## .. ..$ url : chr "https://www.petfinder.com/dog/kodiak-62131013/ia/waterloo/cedar-bend-humane-society-ia36/?referrer_id=4ce20718-"| __truncated__ ## .. ..$ type : chr "Dog" ## .. ..$ species : chr "Dog" ## .. ..$ breeds :List of 4 ## .. .. ..$ primary : chr "Siberian Husky" ## .. .. ..$ secondary: chr "German Shepherd Dog" ## .. .. ..$ mixed : logi TRUE ## .. .. ..$ unknown : logi FALSE ## .. ..$ colors :List of 3 ## .. .. ..$ primary : chr "Brown / Chocolate" ## .. .. ..$ secondary: chr "Gray / Blue / Silver" ## .. .. ..$ tertiary : NULL ## .. ..$ age : chr "Baby" ## .. ..$ gender : chr "Male" ## .. ..$ size : chr "Large" ## .. ..$ coat : chr "Medium" ## .. ..$ attributes :List of 5 ## .. .. ..$ spayed_neutered: logi FALSE ## .. .. ..$ house_trained : logi FALSE ## .. .. ..$ declawed : NULL ## .. .. ..$ special_needs : logi FALSE ## .. .. ..$ shots_current : logi TRUE ## .. ..$ environment :List of 3 ## .. .. ..$ children: logi TRUE ## .. .. ..$ dogs : logi TRUE ## .. .. ..$ cats : logi TRUE ## .. ..$ tags :List of 7 ## .. .. ..$ : chr "Friendly" ## .. .. ..$ : chr "Affectionate" ## .. .. ..$ : chr "Curious" ## .. .. ..$ : chr "Loves kisses" ## .. .. ..$ : chr "Playful" ## .. .. ..$ : chr "Athletic" ## .. .. ..$ : chr "Funny" ## .. ..$ name : chr "Kodiak" ## .. ..$ description : chr "MY STORY: Kodiak came to CBHS as a stray.\n\nAGE: Approximately 3 months old. \n\nHiya friends!\nDo you like going..." ## .. ..$ organization_animal_id: NULL ## .. ..$ photos :List of 3 ## .. .. ..$ :List of 4 ## .. .. .. ..$ small : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131013/1/?bust=1680619470&width=100" ## .. .. .. ..$ medium: chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131013/1/?bust=1680619470&width=300" ## .. .. .. ..$ large : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131013/1/?bust=1680619470&width=600" ## .. .. .. ..$ full : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131013/1/?bust=1680619470" ## .. .. ..$ :List of 4 ## .. .. .. ..$ small : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131013/2/?bust=1680619471&width=100" ## .. .. .. ..$ medium: chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131013/2/?bust=1680619471&width=300" ## .. .. .. ..$ large : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131013/2/?bust=1680619471&width=600" ## .. .. .. ..$ full : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131013/2/?bust=1680619471" ## .. .. ..$ :List of 4 ## .. .. .. ..$ small : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131013/3/?bust=1680619472&width=100" ## .. .. .. ..$ medium: chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131013/3/?bust=1680619472&width=300" ## .. .. .. ..$ large : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131013/3/?bust=1680619472&width=600" ## .. .. .. ..$ full : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131013/3/?bust=1680619472" ## .. ..$ primary_photo_cropped :List of 4 ## .. .. ..$ small : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131013/1/?bust=1680619470&width=300" ## .. .. ..$ medium: chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131013/1/?bust=1680619470&width=450" ## .. .. ..$ large : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131013/1/?bust=1680619470&width=600" ## .. .. ..$ full : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131013/1/?bust=1680619470" ## .. ..$ videos : list() ## .. ..$ status : chr "adoptable" ## .. ..$ status_changed_at : chr "2023-04-04T14:44:34+0000" ## .. ..$ published_at : chr "2023-04-04T14:44:33+0000" ## .. ..$ distance : num 72.3 ## .. ..$ contact :List of 3 ## .. .. ..$ email : chr "adoptioncounselor@cedarbendhumane.org" ## .. .. ..$ phone : chr "(319) 232-6887" ## .. .. ..$ address:List of 6 ## .. .. .. ..$ address1: chr "1166 West Airline Highway" ## .. .. .. ..$ address2: NULL ## .. .. .. ..$ city : chr "Waterloo" ## .. .. .. ..$ state : chr "IA" ## .. .. .. ..$ postcode: chr "50703" ## .. .. .. ..$ country : chr "US" ## .. ..$ _links :List of 3 ## .. .. ..$ self :List of 1 ## .. .. .. ..$ href: chr "/v2/animals/62131013" ## .. .. ..$ type :List of 1 ## .. .. .. ..$ href: chr "/v2/types/dog" ## .. .. ..$ organization:List of 1 ## .. .. .. ..$ href: chr "/v2/organizations/ia36" ## ..$ :List of 26 ## .. ..$ id : int 62130853 ## .. ..$ organization_id : chr "IA36" ## .. ..$ url : chr "https://www.petfinder.com/dog/kirby-62130853/ia/waterloo/cedar-bend-humane-society-ia36/?referrer_id=4ce20718-9"| __truncated__ ## .. ..$ type : chr "Dog" ## .. ..$ species : chr "Dog" ## .. ..$ breeds :List of 4 ## .. .. ..$ primary : chr "Pit Bull Terrier" ## .. .. ..$ secondary: NULL ## .. .. ..$ mixed : logi TRUE ## .. .. ..$ unknown : logi FALSE ## .. ..$ colors :List of 3 ## .. .. ..$ primary : chr "Brindle" ## .. .. ..$ secondary: NULL ## .. .. ..$ tertiary : NULL ## .. ..$ age : chr "Baby" ## .. ..$ gender : chr "Male" ## .. ..$ size : chr "Large" ## .. ..$ coat : chr "Short" ## .. ..$ attributes :List of 5 ## .. .. ..$ spayed_neutered: logi FALSE ## .. .. ..$ house_trained : logi FALSE ## .. .. ..$ declawed : NULL ## .. .. ..$ special_needs : logi FALSE ## .. .. ..$ shots_current : logi TRUE ## .. ..$ environment :List of 3 ## .. .. ..$ children: NULL ## .. .. ..$ dogs : logi TRUE ## .. .. ..$ cats : logi TRUE ## .. ..$ tags :List of 7 ## .. .. ..$ : chr "Friendly" ## .. .. ..$ : chr "Affectionate" ## .. .. ..$ : chr "Curious" ## .. .. ..$ : chr "Loves kisses" ## .. .. ..$ : chr "Playful" ## .. .. ..$ : chr "Athletic" ## .. .. ..$ : chr "Funny" ## .. ..$ name : chr "Kirby" ## .. ..$ description : chr "MY STORY: Kirby came to CBHS as a stray.\n\nAGE: Approximately 3 months old. \n\nHello!\nI'm Kirby!\nI&#"| __truncated__ ## .. ..$ organization_animal_id: NULL ## .. ..$ photos :List of 3 ## .. .. ..$ :List of 4 ## .. .. .. ..$ small : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62130853/2/?bust=1680617895&width=100" ## .. .. .. ..$ medium: chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62130853/2/?bust=1680617895&width=300" ## .. .. .. ..$ large : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62130853/2/?bust=1680617895&width=600" ## .. .. .. ..$ full : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62130853/2/?bust=1680617895" ## .. .. ..$ :List of 4 ## .. .. .. ..$ small : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62130853/1/?bust=1680617894&width=100" ## .. .. .. ..$ medium: chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62130853/1/?bust=1680617894&width=300" ## .. .. .. ..$ large : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62130853/1/?bust=1680617894&width=600" ## .. .. .. ..$ full : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62130853/1/?bust=1680617894" ## .. .. ..$ :List of 4 ## .. .. .. ..$ small : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62130853/3/?bust=1680617896&width=100" ## .. .. .. ..$ medium: chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62130853/3/?bust=1680617896&width=300" ## .. .. .. ..$ large : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62130853/3/?bust=1680617896&width=600" ## .. .. .. ..$ full : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62130853/3/?bust=1680617896" ## .. ..$ primary_photo_cropped :List of 4 ## .. .. ..$ small : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62130853/2/?bust=1680617895&width=300" ## .. .. ..$ medium: chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62130853/2/?bust=1680617895&width=450" ## .. .. ..$ large : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62130853/2/?bust=1680617895&width=600" ## .. .. ..$ full : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62130853/2/?bust=1680617895" ## .. ..$ videos : list() ## .. ..$ status : chr "adoptable" ## .. ..$ status_changed_at : chr "2023-04-04T14:18:20+0000" ## .. ..$ published_at : chr "2023-04-04T14:18:17+0000" ## .. ..$ distance : num 72.3 ## .. ..$ contact :List of 3 ## .. .. ..$ email : chr "adoptioncounselor@cedarbendhumane.org" ## .. .. ..$ phone : chr "(319) 232-6887" ## .. .. ..$ address:List of 6 ## .. .. .. ..$ address1: chr "1166 West Airline Highway" ## .. .. .. ..$ address2: NULL ## .. .. .. ..$ city : chr "Waterloo" ## .. .. .. ..$ state : chr "IA" ## .. .. .. ..$ postcode: chr "50703" ## .. .. .. ..$ country : chr "US" ## .. ..$ _links :List of 3 ## .. .. ..$ self :List of 1 ## .. .. .. ..$ href: chr "/v2/animals/62130853" ## .. .. ..$ type :List of 1 ## .. .. .. ..$ href: chr "/v2/types/dog" ## .. .. ..$ organization:List of 1 ## .. .. .. ..$ href: chr "/v2/organizations/ia36" ## ..$ :List of 26 ## .. ..$ id : int 62130226 ## .. ..$ organization_id : chr "IA220" ## .. ..$ url : chr "https://www.petfinder.com/dog/zoey-62130226/ia/tama/tama-county-humane-society-ia220/?referrer_id=4ce20718-99c0"| __truncated__ ## .. ..$ type : chr "Dog" ## .. ..$ species : chr "Dog" ## .. ..$ breeds :List of 4 ## .. .. ..$ primary : chr "Yorkshire Terrier" ## .. .. ..$ secondary: NULL ## .. .. ..$ mixed : logi FALSE ## .. .. ..$ unknown : logi FALSE ## .. ..$ colors :List of 3 ## .. .. ..$ primary : NULL ## .. .. ..$ secondary: NULL ## .. .. ..$ tertiary : NULL ## .. ..$ age : chr "Young" ## .. ..$ gender : chr "Female" ## .. ..$ size : chr "Small" ## .. ..$ coat : NULL ## .. ..$ attributes :List of 5 ## .. .. ..$ spayed_neutered: logi TRUE ## .. .. ..$ house_trained : logi TRUE ## .. .. ..$ declawed : NULL ## .. .. ..$ special_needs : logi FALSE ## .. .. ..$ shots_current : logi TRUE ## .. ..$ environment :List of 3 ## .. .. ..$ children: logi TRUE ## .. .. ..$ dogs : logi TRUE ## .. .. ..$ cats : logi TRUE ## .. ..$ tags :List of 4 ## .. .. ..$ : chr "Friendly" ## .. .. ..$ : chr "Affectionate" ## .. .. ..$ : chr "Smart" ## .. .. ..$ : chr "Funny" ## .. ..$ name : chr "Zoey" ## .. ..$ description : chr "Meet Zoey\n\nZoey is a one year old Yorkie/Terrier mix. She loves loves people. Zoey has done well with the..." ## .. ..$ organization_animal_id: NULL ## .. ..$ photos :List of 1 ## .. .. ..$ :List of 4 ## .. .. .. ..$ small : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62130226/1/?bust=1680612576&width=100" ## .. .. .. ..$ medium: chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62130226/1/?bust=1680612576&width=300" ## .. .. .. ..$ large : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62130226/1/?bust=1680612576&width=600" ## .. .. .. ..$ full : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62130226/1/?bust=1680612576" ## .. ..$ primary_photo_cropped : NULL ## .. ..$ videos : list() ## .. ..$ status : chr "adoptable" ## .. ..$ status_changed_at : chr "2023-04-04T12:49:39+0000" ## .. ..$ published_at : chr "2023-04-04T12:49:38+0000" ## .. ..$ distance : num 53.6 ## .. ..$ contact :List of 3 ## .. .. ..$ email : chr "tamacountyhumanesociety@yahoo.com" ## .. .. ..$ phone : chr "(641) 481-7001" ## .. .. ..$ address:List of 6 ## .. .. .. ..$ address1: chr "1406 E 5th Street" ## .. .. .. ..$ address2: NULL ## .. .. .. ..$ city : chr "Tama" ## .. .. .. ..$ state : chr "IA" ## .. .. .. ..$ postcode: chr "52339" ## .. .. .. ..$ country : chr "US" ## .. ..$ _links :List of 3 ## .. .. ..$ self :List of 1 ## .. .. .. ..$ href: chr "/v2/animals/62130226" ## .. .. ..$ type :List of 1 ## .. .. .. ..$ href: chr "/v2/types/dog" ## .. .. ..$ organization:List of 1 ## .. .. .. ..$ href: chr "/v2/organizations/ia220" ## ..$ :List of 26 ## .. ..$ id : int 62118586 ## .. ..$ organization_id : chr "IA172" ## .. ..$ url : chr "https://www.petfinder.com/dog/tinky-62118586/ia/west-des-moines/furry-friends-refuge-ia172/?referrer_id=4ce2071"| __truncated__ ## .. ..$ type : chr "Dog" ## .. ..$ species : chr "Dog" ## .. ..$ breeds :List of 4 ## .. .. ..$ primary : chr "Husky" ## .. .. ..$ secondary: NULL ## .. .. ..$ mixed : logi FALSE ## .. .. ..$ unknown : logi FALSE ## .. ..$ colors :List of 3 ## .. .. ..$ primary : chr "Black" ## .. .. ..$ secondary: NULL ## .. .. ..$ tertiary : NULL ## .. ..$ age : chr "Adult" ## .. ..$ gender : chr "Female" ## .. ..$ size : chr "Medium" ## .. ..$ coat : NULL ## .. ..$ attributes :List of 5 ## .. .. ..$ spayed_neutered: logi TRUE ## .. .. ..$ house_trained : logi FALSE ## .. .. ..$ declawed : NULL ## .. .. ..$ special_needs : logi FALSE ## .. .. ..$ shots_current : logi TRUE ## .. ..$ environment :List of 3 ## .. .. ..$ children: NULL ## .. .. ..$ dogs : NULL ## .. .. ..$ cats : NULL ## .. ..$ tags : list() ## .. ..$ name : chr "Tinky" ## .. ..$ description : NULL ## .. ..$ organization_animal_id: chr "FFS-A-14544" ## .. ..$ photos : list() ## .. ..$ primary_photo_cropped : NULL ## .. ..$ videos : list() ## .. ..$ status : chr "adoptable" ## .. ..$ status_changed_at : chr "2023-04-04T00:40:27+0000" ## .. ..$ published_at : chr "2023-04-04T00:40:26+0000" ## .. ..$ distance : num 33.4 ## .. ..$ contact :List of 3 ## .. .. ..$ email : chr "adoptions@furryfriendsrefuge.org" ## .. .. ..$ phone : chr "(515) 222-0009" ## .. .. ..$ address:List of 6 ## .. .. .. ..$ address1: chr "3505 Mills Civic Parkway" ## .. .. .. ..$ address2: NULL ## .. .. .. ..$ city : chr "West Des Moines" ## .. .. .. ..$ state : chr "IA" ## .. .. .. ..$ postcode: chr "50265" ## .. .. .. ..$ country : chr "US" ## .. ..$ _links :List of 3 ## .. .. ..$ self :List of 1 ## .. .. .. ..$ href: chr "/v2/animals/62118586" ## .. .. ..$ type :List of 1 ## .. .. .. ..$ href: chr "/v2/types/dog" ## .. .. ..$ organization:List of 1 ## .. .. .. ..$ href: chr "/v2/organizations/ia172" ## ..$ :List of 26 ## .. ..$ id : int 62073733 ## .. ..$ organization_id : chr "IA242" ## .. ..$ url : chr "https://www.petfinder.com/dog/bear-62073733/ia/independence/wildthunder-w-dot-ar-dot-s-ia242/?referrer_id=4ce20"| __truncated__ ## .. ..$ type : chr "Dog" ## .. ..$ species : chr "Dog" ## .. ..$ breeds :List of 4 ## .. .. ..$ primary : chr "American Staffordshire Terrier" ## .. .. ..$ secondary: NULL ## .. .. ..$ mixed : logi TRUE ## .. .. ..$ unknown : logi FALSE ## .. ..$ colors :List of 3 ## .. .. ..$ primary : chr "Yellow / Tan / Blond / Fawn" ## .. .. ..$ secondary: NULL ## .. .. ..$ tertiary : NULL ## .. ..$ age : chr "Adult" ## .. ..$ gender : chr "Male" ## .. ..$ size : chr "Large" ## .. ..$ coat : NULL ## .. ..$ attributes :List of 5 ## .. .. ..$ spayed_neutered: logi TRUE ## .. .. ..$ house_trained : logi TRUE ## .. .. ..$ declawed : NULL ## .. .. ..$ special_needs : logi FALSE ## .. .. ..$ shots_current : logi TRUE ## .. ..$ environment :List of 3 ## .. .. ..$ children: logi TRUE ## .. .. ..$ dogs : logi TRUE ## .. .. ..$ cats : NULL ## .. ..$ tags :List of 5 ## .. .. ..$ : chr "Friendly" ## .. .. ..$ : chr "Affectionate" ## .. .. ..$ : chr "Playful" ## .. .. ..$ : chr "Smart" ## .. .. ..$ : chr "Funny" ## .. ..$ name : chr "Bear" ## .. ..$ description : chr "Bear is a sweet 6yr old pittie who came to us from an owner who no longer wanted to care..." ## .. ..$ organization_animal_id: NULL ## .. ..$ photos :List of 3 ## .. .. ..$ :List of 4 ## .. .. .. ..$ small : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62073733/1/?bust=1680530960&width=100" ## .. .. .. ..$ medium: chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62073733/1/?bust=1680530960&width=300" ## .. .. .. ..$ large : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62073733/1/?bust=1680530960&width=600" ## .. .. .. ..$ full : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62073733/1/?bust=1680530960" ## .. .. ..$ :List of 4 ## .. .. .. ..$ small : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62073733/2/?bust=1680567042&width=100" ## .. .. .. ..$ medium: chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62073733/2/?bust=1680567042&width=300" ## .. .. .. ..$ large : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62073733/2/?bust=1680567042&width=600" ## .. .. .. ..$ full : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62073733/2/?bust=1680567042" ## .. .. ..$ :List of 4 ## .. .. .. ..$ small : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62073733/3/?bust=1680567043&width=100" ## .. .. .. ..$ medium: chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62073733/3/?bust=1680567043&width=300" ## .. .. .. ..$ large : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62073733/3/?bust=1680567043&width=600" ## .. .. .. ..$ full : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62073733/3/?bust=1680567043" ## .. ..$ primary_photo_cropped :List of 4 ## .. .. ..$ small : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62073733/1/?bust=1680530960&width=300" ## .. .. ..$ medium: chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62073733/1/?bust=1680530960&width=450" ## .. .. ..$ large : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62073733/1/?bust=1680530960&width=600" ## .. .. ..$ full : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62073733/1/?bust=1680530960" ## .. ..$ videos : list() ## .. ..$ status : chr "adoptable" ## .. ..$ status_changed_at : chr "2023-04-04T00:10:47+0000" ## .. ..$ published_at : chr "2023-04-04T00:10:46+0000" ## .. ..$ distance : num 92.4 ## .. ..$ contact :List of 3 ## .. .. ..$ email : chr "adoptions@wildthunderwars.org" ## .. .. ..$ phone : chr "(319) 961-3352" ## .. .. ..$ address:List of 6 ## .. .. .. ..$ address1: NULL ## .. .. .. ..$ address2: NULL ## .. .. .. ..$ city : chr "Independence" ## .. .. .. ..$ state : chr "IA" ## .. .. .. ..$ postcode: chr "50644" ## .. .. .. ..$ country : chr "US" ## .. ..$ _links :List of 3 ## .. .. ..$ self :List of 1 ## .. .. .. ..$ href: chr "/v2/animals/62073733" ## .. .. ..$ type :List of 1 ## .. .. .. ..$ href: chr "/v2/types/dog" ## .. .. ..$ organization:List of 1 ## .. .. .. ..$ href: chr "/v2/organizations/ia242" ## ..$ :List of 26 ## .. ..$ id : int 62081049 ## .. ..$ organization_id : chr "IA146" ## .. ..$ url : chr "https://www.petfinder.com/dog/blaze-62081049/ia/carroll/animal-rescue-of-carroll-ia146/?referrer_id=4ce20718-99"| __truncated__ ## .. ..$ type : chr "Dog" ## .. ..$ species : chr "Dog" ## .. ..$ breeds :List of 4 ## .. .. ..$ primary : chr "Black Labrador Retriever" ## .. .. ..$ secondary: chr "Mixed Breed" ## .. .. ..$ mixed : logi TRUE ## .. .. ..$ unknown : logi FALSE ## .. ..$ colors :List of 3 ## .. .. ..$ primary : chr "Black" ## .. .. ..$ secondary: NULL ## .. .. ..$ tertiary : NULL ## .. ..$ age : chr "Adult" ## .. ..$ gender : chr "Male" ## .. ..$ size : chr "Large" ## .. ..$ coat : NULL ## .. ..$ attributes :List of 5 ## .. .. ..$ spayed_neutered: logi TRUE ## .. .. ..$ house_trained : logi FALSE ## .. .. ..$ declawed : NULL ## .. .. ..$ special_needs : logi FALSE ## .. .. ..$ shots_current : logi TRUE ## .. ..$ environment :List of 3 ## .. .. ..$ children: NULL ## .. .. ..$ dogs : NULL ## .. .. ..$ cats : NULL ## .. ..$ tags : list() ## .. ..$ name : chr "Blaze" ## .. ..$ description : NULL ## .. ..$ organization_animal_id: chr "ARC-A-4146" ## .. ..$ photos :List of 1 ## .. .. ..$ :List of 4 ## .. .. .. ..$ small : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62081049/1/?bust=1680562217&width=100" ## .. .. .. ..$ medium: chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62081049/1/?bust=1680562217&width=300" ## .. .. .. ..$ large : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62081049/1/?bust=1680562217&width=600" ## .. .. .. ..$ full : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62081049/1/?bust=1680562217" ## .. ..$ primary_photo_cropped :List of 4 ## .. .. ..$ small : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62081049/1/?bust=1680562217&width=300" ## .. .. ..$ medium: chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62081049/1/?bust=1680562217&width=450" ## .. .. ..$ large : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62081049/1/?bust=1680562217&width=600" ## .. .. ..$ full : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62081049/1/?bust=1680562217" ## .. ..$ videos : list() ## .. ..$ status : chr "adoptable" ## .. ..$ status_changed_at : chr "2023-04-03T22:40:10+0000" ## .. ..$ published_at : chr "2023-04-03T22:40:08+0000" ## .. ..$ distance : num 65 ## .. ..$ contact :List of 3 ## .. .. ..$ email : chr "animalrescueofcarroll@yahoo.com" ## .. .. ..$ phone : chr "(712) 790-9116" ## .. .. ..$ address:List of 6 ## .. .. .. ..$ address1: chr "1721 E 10th Street" ## .. .. .. ..$ address2: chr "PO Box 393" ## .. .. .. ..$ city : chr "Carroll" ## .. .. .. ..$ state : chr "IA" ## .. .. .. ..$ postcode: chr "51401" ## .. .. .. ..$ country : chr "US" ## .. ..$ _links :List of 3 ## .. .. ..$ self :List of 1 ## .. .. .. ..$ href: chr "/v2/animals/62081049" ## .. .. ..$ type :List of 1 ## .. .. .. ..$ href: chr "/v2/types/dog" ## .. .. ..$ organization:List of 1 ## .. .. .. ..$ href: chr "/v2/organizations/ia146" ## ..$ :List of 26 ## .. ..$ id : int 62081025 ## .. ..$ organization_id : chr "IA36" ## .. ..$ url : chr "https://www.petfinder.com/dog/barry-150-dollars-adoption-fee-until-april-8-62081025/ia/waterloo/cedar-bend-huma"| __truncated__ ## .. ..$ type : chr "Dog" ## .. ..$ species : chr "Dog" ## .. ..$ breeds :List of 4 ## .. .. ..$ primary : chr "Chihuahua" ## .. .. ..$ secondary: chr "Terrier" ## .. .. ..$ mixed : logi TRUE ## .. .. ..$ unknown : logi FALSE ## .. ..$ colors :List of 3 ## .. .. ..$ primary : chr "Black" ## .. .. ..$ secondary: chr "Brown / Chocolate" ## .. .. ..$ tertiary : NULL ## .. ..$ age : chr "Adult" ## .. ..$ gender : chr "Male" ## .. ..$ size : chr "Small" ## .. ..$ coat : chr "Short" ## .. ..$ attributes :List of 5 ## .. .. ..$ spayed_neutered: logi FALSE ## .. .. ..$ house_trained : logi FALSE ## .. .. ..$ declawed : NULL ## .. .. ..$ special_needs : logi FALSE ## .. .. ..$ shots_current : logi TRUE ## .. ..$ environment :List of 3 ## .. .. ..$ children: NULL ## .. .. ..$ dogs : logi TRUE ## .. .. ..$ cats : NULL ## .. ..$ tags :List of 6 ## .. .. ..$ : chr "Friendly" ## .. .. ..$ : chr "Affectionate" ## .. .. ..$ : chr "Curious" ## .. .. ..$ : chr "Couch potato" ## .. .. ..$ : chr "Loves kisses" ## .. .. ..$ : chr "Gentle" ## .. ..$ name : chr "Barry - $150 adoption fee until April 8" ## .. ..$ description : chr "MY STORY: Barry came from a partnering rescue organization. \n\nAGE: Approximately 6 years old. \n\nHi, I'"| __truncated__ ## .. ..$ organization_animal_id: NULL ## .. ..$ photos :List of 3 ## .. .. ..$ :List of 4 ## .. .. .. ..$ small : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62081025/3/?bust=1680561444&width=100" ## .. .. .. ..$ medium: chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62081025/3/?bust=1680561444&width=300" ## .. .. .. ..$ large : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62081025/3/?bust=1680561444&width=600" ## .. .. .. ..$ full : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62081025/3/?bust=1680561444" ## .. .. ..$ :List of 4 ## .. .. .. ..$ small : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62081025/1/?bust=1680561442&width=100" ## .. .. .. ..$ medium: chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62081025/1/?bust=1680561442&width=300" ## .. .. .. ..$ large : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62081025/1/?bust=1680561442&width=600" ## .. .. .. ..$ full : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62081025/1/?bust=1680561442" ## .. .. ..$ :List of 4 ## .. .. .. ..$ small : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62081025/2/?bust=1680561443&width=100" ## .. .. .. ..$ medium: chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62081025/2/?bust=1680561443&width=300" ## .. .. .. ..$ large : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62081025/2/?bust=1680561443&width=600" ## .. .. .. ..$ full : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62081025/2/?bust=1680561443" ## .. ..$ primary_photo_cropped :List of 4 ## .. .. ..$ small : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62081025/3/?bust=1680561444&width=300" ## .. .. ..$ medium: chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62081025/3/?bust=1680561444&width=450" ## .. .. ..$ large : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62081025/3/?bust=1680561444&width=600" ## .. .. ..$ full : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62081025/3/?bust=1680561444" ## .. ..$ videos : list() ## .. ..$ status : chr "adoptable" ## .. ..$ status_changed_at : chr "2023-04-03T22:37:26+0000" ## .. ..$ published_at : chr "2023-04-03T22:37:25+0000" ## .. ..$ distance : num 72.3 ## .. ..$ contact :List of 3 ## .. .. ..$ email : chr "adoptioncounselor@cedarbendhumane.org" ## .. .. ..$ phone : chr "(319) 232-6887" ## .. .. ..$ address:List of 6 ## .. .. .. ..$ address1: chr "1166 West Airline Highway" ## .. .. .. ..$ address2: NULL ## .. .. .. ..$ city : chr "Waterloo" ## .. .. .. ..$ state : chr "IA" ## .. .. .. ..$ postcode: chr "50703" ## .. .. .. ..$ country : chr "US" ## .. ..$ _links :List of 3 ## .. .. ..$ self :List of 1 ## .. .. .. ..$ href: chr "/v2/animals/62081025" ## .. .. ..$ type :List of 1 ## .. .. .. ..$ href: chr "/v2/types/dog" ## .. .. ..$ organization:List of 1 ## .. .. .. ..$ href: chr "/v2/organizations/ia36" ## ..$ :List of 26 ## .. ..$ id : int 62080810 ## .. ..$ organization_id : chr "IA264" ## .. ..$ url : chr "https://www.petfinder.com/dog/juliette-62080810/ia/humboldt/humboldt-county-animal-shelter-ia264/?referrer_id=4"| __truncated__ ## .. ..$ type : chr "Dog" ## .. ..$ species : chr "Dog" ## .. ..$ breeds :List of 4 ## .. .. ..$ primary : chr "Rat Terrier" ## .. .. ..$ secondary: chr "Husky" ## .. .. ..$ mixed : logi TRUE ## .. .. ..$ unknown : logi FALSE ## .. ..$ colors :List of 3 ## .. .. ..$ primary : chr "Bicolor" ## .. .. ..$ secondary: chr "Brown / Chocolate" ## .. .. ..$ tertiary : chr "White / Cream" ## .. ..$ age : chr "Baby" ## .. ..$ gender : chr "Female" ## .. ..$ size : chr "Medium" ## .. ..$ coat : NULL ## .. ..$ attributes :List of 5 ## .. .. ..$ spayed_neutered: logi TRUE ## .. .. ..$ house_trained : logi FALSE ## .. .. ..$ declawed : NULL ## .. .. ..$ special_needs : logi FALSE ## .. .. ..$ shots_current : logi TRUE ## .. ..$ environment :List of 3 ## .. .. ..$ children: logi TRUE ## .. .. ..$ dogs : logi TRUE ## .. .. ..$ cats : NULL ## .. ..$ tags :List of 9 ## .. .. ..$ : chr "Friendly" ## .. .. ..$ : chr "Affectionate" ## .. .. ..$ : chr "Playful" ## .. .. ..$ : chr "Smart" ## .. .. ..$ : chr "Brave" ## .. .. ..$ : chr "Curious" ## .. .. ..$ : chr "Funny" ## .. .. ..$ : chr "Athletic" ## .. .. ..$ : chr "Loves kisses" ## .. ..$ name : chr "Juliette " ## .. ..$ description : chr "This sweet little fire cracker would love to lick your face and show you how much she loves you while..." ## .. ..$ organization_animal_id: NULL ## .. ..$ photos :List of 2 ## .. .. ..$ :List of 4 ## .. .. .. ..$ small : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62080810/1/?bust=1680560804&width=100" ## .. .. .. ..$ medium: chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62080810/1/?bust=1680560804&width=300" ## .. .. .. ..$ large : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62080810/1/?bust=1680560804&width=600" ## .. .. .. ..$ full : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62080810/1/?bust=1680560804" ## .. .. ..$ :List of 4 ## .. .. .. ..$ small : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62080810/2/?bust=1680560810&width=100" ## .. .. .. ..$ medium: chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62080810/2/?bust=1680560810&width=300" ## .. .. .. ..$ large : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62080810/2/?bust=1680560810&width=600" ## .. .. .. ..$ full : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62080810/2/?bust=1680560810" ## .. ..$ primary_photo_cropped :List of 4 ## .. .. ..$ small : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62080810/1/?bust=1680560804&width=300" ## .. .. ..$ medium: chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62080810/1/?bust=1680560804&width=450" ## .. .. ..$ large : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62080810/1/?bust=1680560804&width=600" ## .. .. ..$ full : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62080810/1/?bust=1680560804" ## .. ..$ videos :List of 1 ## .. .. ..$ :List of 1 ## .. .. .. ..$ embed: chr "<iframe title=\"Video\" frameborder=\"0\" allowfullscreen webkitallowfullscreen mozallowfullscreen msallowfulls"| __truncated__ ## .. ..$ status : chr "adoptable" ## .. ..$ status_changed_at : chr "2023-04-03T22:26:53+0000" ## .. ..$ published_at : chr "2023-04-03T22:26:51+0000" ## .. ..$ distance : num 56.7 ## .. ..$ contact :List of 3 ## .. .. ..$ email : chr "Moffitt.adopt@gmail.com" ## .. .. ..$ phone : chr "(515) 604-6242" ## .. .. ..$ address:List of 6 ## .. .. .. ..$ address1: NULL ## .. .. .. ..$ address2: NULL ## .. .. .. ..$ city : chr "Humboldt" ## .. .. .. ..$ state : chr "IA" ## .. .. .. ..$ postcode: chr "50548" ## .. .. .. ..$ country : chr "US" ## .. ..$ _links :List of 3 ## .. .. ..$ self :List of 1 ## .. .. .. ..$ href: chr "/v2/animals/62080810" ## .. .. ..$ type :List of 1 ## .. .. .. ..$ href: chr "/v2/types/dog" ## .. .. ..$ organization:List of 1 ## .. .. .. ..$ href: chr "/v2/organizations/ia264" ## ..$ :List of 26 ## .. ..$ id : int 62080339 ## .. ..$ organization_id : chr "IA172" ## .. ..$ url : chr "https://www.petfinder.com/dog/prancer-62080339/ia/west-des-moines/furry-friends-refuge-ia172/?referrer_id=4ce20"| __truncated__ ## .. ..$ type : chr "Dog" ## .. ..$ species : chr "Dog" ## .. ..$ breeds :List of 4 ## .. .. ..$ primary : chr "Black Labrador Retriever" ## .. .. ..$ secondary: chr "Bichon Frise" ## .. .. ..$ mixed : logi TRUE ## .. .. ..$ unknown : logi FALSE ## .. ..$ colors :List of 3 ## .. .. ..$ primary : chr "Black" ## .. .. ..$ secondary: NULL ## .. .. ..$ tertiary : NULL ## .. ..$ age : chr "Adult" ## .. ..$ gender : chr "Female" ## .. ..$ size : chr "Medium" ## .. ..$ coat : NULL ## .. ..$ attributes :List of 5 ## .. .. ..$ spayed_neutered: logi TRUE ## .. .. ..$ house_trained : logi FALSE ## .. .. ..$ declawed : NULL ## .. .. ..$ special_needs : logi FALSE ## .. .. ..$ shots_current : logi TRUE ## .. ..$ environment :List of 3 ## .. .. ..$ children: NULL ## .. .. ..$ dogs : logi FALSE ## .. .. ..$ cats : NULL ## .. ..$ tags :List of 2 ## .. .. ..$ : chr "Home without cats" ## .. .. ..$ : chr "Single Dog Home" ## .. ..$ name : chr "Prancer" ## .. ..$ description : NULL ## .. ..$ organization_animal_id: chr "FFS-A-14660" ## .. ..$ photos :List of 1 ## .. .. ..$ :List of 4 ## .. .. .. ..$ small : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62080339/1/?bust=1680562216&width=100" ## .. .. .. ..$ medium: chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62080339/1/?bust=1680562216&width=300" ## .. .. .. ..$ large : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62080339/1/?bust=1680562216&width=600" ## .. .. .. ..$ full : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62080339/1/?bust=1680562216" ## .. ..$ primary_photo_cropped :List of 4 ## .. .. ..$ small : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62080339/1/?bust=1680562216&width=300" ## .. .. ..$ medium: chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62080339/1/?bust=1680562216&width=450" ## .. .. ..$ large : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62080339/1/?bust=1680562216&width=600" ## .. .. ..$ full : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62080339/1/?bust=1680562216" ## .. ..$ videos : list() ## .. ..$ status : chr "adoptable" ## .. ..$ status_changed_at : chr "2023-04-03T21:41:21+0000" ## .. ..$ published_at : chr "2023-04-03T21:41:19+0000" ## .. ..$ distance : num 33.4 ## .. ..$ contact :List of 3 ## .. .. ..$ email : chr "adoptions@furryfriendsrefuge.org" ## .. .. ..$ phone : chr "(515) 222-0009" ## .. .. ..$ address:List of 6 ## .. .. .. ..$ address1: chr "3505 Mills Civic Parkway" ## .. .. .. ..$ address2: NULL ## .. .. .. ..$ city : chr "West Des Moines" ## .. .. .. ..$ state : chr "IA" ## .. .. .. ..$ postcode: chr "50265" ## .. .. .. ..$ country : chr "US" ## .. ..$ _links :List of 3 ## .. .. ..$ self :List of 1 ## .. .. .. ..$ href: chr "/v2/animals/62080339" ## .. .. ..$ type :List of 1 ## .. .. .. ..$ href: chr "/v2/types/dog" ## .. .. ..$ organization:List of 1 ## .. .. .. ..$ href: chr "/v2/organizations/ia172" ## ..$ :List of 26 ## .. ..$ id : int 62079842 ## .. ..$ organization_id : chr "IA264" ## .. ..$ url : chr "https://www.petfinder.com/dog/blue-62079842/ia/humboldt/humboldt-county-animal-shelter-ia264/?referrer_id=4ce20"| __truncated__ ## .. ..$ type : chr "Dog" ## .. ..$ species : chr "Dog" ## .. ..$ breeds :List of 4 ## .. .. ..$ primary : chr "Great Dane" ## .. .. ..$ secondary: chr "Boxer" ## .. .. ..$ mixed : logi TRUE ## .. .. ..$ unknown : logi FALSE ## .. ..$ colors :List of 3 ## .. .. ..$ primary : chr "White / Cream" ## .. .. ..$ secondary: chr "Tricolor (Brown, Black, & White)" ## .. .. ..$ tertiary : NULL ## .. ..$ age : chr "Young" ## .. ..$ gender : chr "Male" ## .. ..$ size : chr "Extra Large" ## .. ..$ coat : chr "Short" ## .. ..$ attributes :List of 5 ## .. .. ..$ spayed_neutered: logi TRUE ## .. .. ..$ house_trained : logi TRUE ## .. .. ..$ declawed : NULL ## .. .. ..$ special_needs : logi FALSE ## .. .. ..$ shots_current : logi TRUE ## .. ..$ environment :List of 3 ## .. .. ..$ children: NULL ## .. .. ..$ dogs : NULL ## .. .. ..$ cats : NULL ## .. ..$ tags : list() ## .. ..$ name : chr "Blue" ## .. ..$ description : chr "Blue is a special boy that needs someone to show him that he’s safe and loved and build his confidence...." ## .. ..$ organization_animal_id: NULL ## .. ..$ photos :List of 2 ## .. .. ..$ :List of 4 ## .. .. .. ..$ small : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62079842/1/?bust=1680555032&width=100" ## .. .. .. ..$ medium: chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62079842/1/?bust=1680555032&width=300" ## .. .. .. ..$ large : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62079842/1/?bust=1680555032&width=600" ## .. .. .. ..$ full : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62079842/1/?bust=1680555032" ## .. .. ..$ :List of 4 ## .. .. .. ..$ small : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62079842/2/?bust=1680555033&width=100" ## .. .. .. ..$ medium: chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62079842/2/?bust=1680555033&width=300" ## .. .. .. ..$ large : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62079842/2/?bust=1680555033&width=600" ## .. .. .. ..$ full : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62079842/2/?bust=1680555033" ## .. ..$ primary_photo_cropped :List of 4 ## .. .. ..$ small : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62079842/1/?bust=1680555032&width=300" ## .. .. ..$ medium: chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62079842/1/?bust=1680555032&width=450" ## .. .. ..$ large : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62079842/1/?bust=1680555032&width=600" ## .. .. ..$ full : chr "https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62079842/1/?bust=1680555032" ## .. ..$ videos : list() ## .. ..$ status : chr "adoptable" ## .. ..$ status_changed_at : chr "2023-04-03T20:50:35+0000" ## .. ..$ published_at : chr "2023-04-03T20:50:34+0000" ## .. ..$ distance : num 56.7 ## .. ..$ contact :List of 3 ## .. .. ..$ email : chr "Moffitt.adopt@gmail.com" ## .. .. ..$ phone : chr "(515) 604-6242" ## .. .. ..$ address:List of 6 ## .. .. .. ..$ address1: NULL ## .. .. .. ..$ address2: NULL ## .. .. .. ..$ city : chr "Humboldt" ## .. .. .. ..$ state : chr "IA" ## .. .. .. ..$ postcode: chr "50548" ## .. .. .. ..$ country : chr "US" ## .. ..$ _links :List of 3 ## .. .. ..$ self :List of 1 ## .. .. .. ..$ href: chr "/v2/animals/62079842" ## .. .. ..$ type :List of 1 ## .. .. .. ..$ href: chr "/v2/types/dog" ## .. .. ..$ organization:List of 1 ## .. .. .. ..$ href: chr "/v2/organizations/ia264" ## $ pagination:List of 5 ## ..$ count_per_page: int 20 ## ..$ total_count : int 679 ## ..$ current_page : int 1 ## ..$ total_pages : int 34 ## ..$ _links :List of 1 ## .. ..$ next:List of 1 ## .. .. ..$ href: chr "/v2/animals?location=50010&type=dog&page=2" ``` --- class:inverse ## Your Turn The [ames-dogs.Rdata](https://bit.ly/3tU4dKG) file is the result from the query on the last slide. ```r url_dogs <- "https://bit.ly/3ZsuIUc" # download the file to your drive download.file(url_dogs, destfile = "ames-dogs.Rdata", mode="wb") load("ames-dogs.Rdata") ``` 1. For the dogs returned by the API, assemble a data frame with their name, age, sex, breed(s), and shelter ID. 2. For each dog, can you assemble a list of picture links? (Hint: use list columns) --- ## Your Turn Solutions ```r library(xml2) getBreedInfo <- function(breed) { res <- "unknown" if (breed$unknown) return(res) res <- breed$primary if (!is.null(breed$secondary)) res <- paste(res, breed$secondary, sep=", ") if (breed$mixed) res <- paste(res, "Mix", sep=", ") return(res) } dog_to_df <- function(x, verbose=FALSE) { if (verbose) { cat(x$name) cat("\n") } tibble( name = x$name, breed = getBreedInfo(x$breeds), age = x$age, sex = x$sex, id = x$id, shelterID = x$shelterId, pics = list(x$photos) ) } ames_dog_df <- ames_dogs$animals %>% purrr::map_df(dog_to_df) ``` --- ## Your Turn Solutions ```r ames_dog_df ``` ``` ## # A tibble: 20 × 5 ## name breed age id pics ## <chr> <chr> <chr> <int> <list> ## 1 "Bo" Aust… Adult 6.21e7 <list> ## 2 "Minerva" Germ… Young 6.21e7 <list> ## 3 "Tucker" Shep… Young 6.21e7 <list> ## 4 "Pubby" Pit … Young 6.21e7 <list> ## 5 "Diesel" Rott… Baby 6.21e7 <list> ## 6 "Ghost" Labr… Young 6.21e7 <list> ## 7 "Frankie" Germ… Young 6.21e7 <list> ## 8 "Gambler" Aust… Adult 6.21e7 <list> ## 9 "Lucille " Aust… Adult 6.21e7 <list> ## 10 "Dakota" Catt… Adult 6.21e7 <list> ## 11 "Kodiak" Sibe… Baby 6.21e7 <list> ## 12 "Kirby" Pit … Baby 6.21e7 <list> ## 13 "Zoey" York… Young 6.21e7 <list> ## 14 "Tinky" Husky Adult 6.21e7 <list> ## 15 "Bear" Amer… Adult 6.21e7 <list> ## 16 "Blaze" Blac… Adult 6.21e7 <list> ## 17 "Barry - $150 adoption fee unt… Chih… Adult 6.21e7 <list> ## 18 "Juliette " Rat … Baby 6.21e7 <list> ## 19 "Prancer" Blac… Adult 6.21e7 <list> ## 20 "Blue" Grea… Young 6.21e7 <list> ``` --- ## Your Turn Solutions ```r purrr::map_chr(ames_dog_df$pics, .f = function(x) { if (length(x) == 0) return(NA) x[[1]]$full }) %>% na.omit() %>% knitr::include_graphics() ``` <img src="https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62134825/1/?bust=1680632275" width="10%" /><img src="https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62133004/1/?bust=1680633569" width="10%" /><img src="https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62132676/1/?bust=1680632546" width="10%" /><img src="https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131790/1/?bust=1680623359" width="10%" /><img src="https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131338/1/?bust=1680624708" width="10%" /><img src="https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62131013/1/?bust=1680619470" width="10%" /><img src="https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62130853/2/?bust=1680617895" width="10%" /><img src="https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62130226/1/?bust=1680612576" width="10%" /><img src="https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62073733/1/?bust=1680530960" width="10%" /><img src="https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62081049/1/?bust=1680562217" width="10%" /><img src="https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62081025/3/?bust=1680561444" width="10%" /><img src="https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62080810/1/?bust=1680560804" width="10%" /><img src="https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62080339/1/?bust=1680562216" width="10%" /><img src="https://dl5zpyw5k3jeb.cloudfront.net/photos/pets/62079842/1/?bust=1680555032" width="10%" /> --- ## Whether the weather or weather the weather? Read through the [NOAA API](https://www.ncdc.noaa.gov/cdo-web/webservices/v2) documentation and try out queries in the web browser to check how to read responses correctly. ```r jsonlite::read_json("https://api.weather.gov/points/41.9906,-93.6189") ``` ``` ## $`@context` ## $`@context`[[1]] ## [1] "https://geojson.org/geojson-ld/geojson-context.jsonld" ## ## $`@context`[[2]] ## $`@context`[[2]]$`@version` ## [1] "1.1" ## ## $`@context`[[2]]$wx ## [1] "https://api.weather.gov/ontology#" ## ## $`@context`[[2]]$s ## [1] "https://schema.org/" ## ## $`@context`[[2]]$geo ## [1] "http://www.opengis.net/ont/geosparql#" ## ## $`@context`[[2]]$unit ## [1] "http://codes.wmo.int/common/unit/" ## ## $`@context`[[2]]$`@vocab` ## [1] "https://api.weather.gov/ontology#" ## ## $`@context`[[2]]$geometry ## $`@context`[[2]]$geometry$`@id` ## [1] "s:GeoCoordinates" ## ## $`@context`[[2]]$geometry$`@type` ## [1] "geo:wktLiteral" ## ## ## $`@context`[[2]]$city ## [1] "s:addressLocality" ## ## $`@context`[[2]]$state ## [1] "s:addressRegion" ## ## $`@context`[[2]]$distance ## $`@context`[[2]]$distance$`@id` ## [1] "s:Distance" ## ## $`@context`[[2]]$distance$`@type` ## [1] "s:QuantitativeValue" ## ## ## $`@context`[[2]]$bearing ## $`@context`[[2]]$bearing$`@type` ## [1] "s:QuantitativeValue" ## ## ## $`@context`[[2]]$value ## $`@context`[[2]]$value$`@id` ## [1] "s:value" ## ## ## $`@context`[[2]]$unitCode ## $`@context`[[2]]$unitCode$`@id` ## [1] "s:unitCode" ## ## $`@context`[[2]]$unitCode$`@type` ## [1] "@id" ## ## ## $`@context`[[2]]$forecastOffice ## $`@context`[[2]]$forecastOffice$`@type` ## [1] "@id" ## ## ## $`@context`[[2]]$forecastGridData ## $`@context`[[2]]$forecastGridData$`@type` ## [1] "@id" ## ## ## $`@context`[[2]]$publicZone ## $`@context`[[2]]$publicZone$`@type` ## [1] "@id" ## ## ## $`@context`[[2]]$county ## $`@context`[[2]]$county$`@type` ## [1] "@id" ## ## ## ## ## $id ## [1] "https://api.weather.gov/points/41.9906,-93.6189" ## ## $type ## [1] "Feature" ## ## $geometry ## $geometry$type ## [1] "Point" ## ## $geometry$coordinates ## $geometry$coordinates[[1]] ## [1] -93.6189 ## ## $geometry$coordinates[[2]] ## [1] 41.9906 ## ## ## ## $properties ## $properties$`@id` ## [1] "https://api.weather.gov/points/41.9906,-93.6189" ## ## $properties$`@type` ## [1] "wx:Point" ## ## $properties$cwa ## [1] "DMX" ## ## $properties$forecastOffice ## [1] "https://api.weather.gov/offices/DMX" ## ## $properties$gridId ## [1] "DMX" ## ## $properties$gridX ## [1] 73 ## ## $properties$gridY ## [1] 68 ## ## $properties$forecast ## [1] "https://api.weather.gov/gridpoints/DMX/73,68/forecast" ## ## $properties$forecastHourly ## [1] "https://api.weather.gov/gridpoints/DMX/73,68/forecast/hourly" ## ## $properties$forecastGridData ## [1] "https://api.weather.gov/gridpoints/DMX/73,68" ## ## $properties$observationStations ## [1] "https://api.weather.gov/gridpoints/DMX/73,68/stations" ## ## $properties$relativeLocation ## $properties$relativeLocation$type ## [1] "Feature" ## ## $properties$relativeLocation$geometry ## $properties$relativeLocation$geometry$type ## [1] "Point" ## ## $properties$relativeLocation$geometry$coordinates ## $properties$relativeLocation$geometry$coordinates[[1]] ## [1] -93.62775 ## ## $properties$relativeLocation$geometry$coordinates[[2]] ## [1] 42.02602 ## ## ## ## $properties$relativeLocation$properties ## $properties$relativeLocation$properties$city ## [1] "Ames" ## ## $properties$relativeLocation$properties$state ## [1] "IA" ## ## $properties$relativeLocation$properties$distance ## $properties$relativeLocation$properties$distance$unitCode ## [1] "wmoUnit:m" ## ## $properties$relativeLocation$properties$distance$value ## [1] 4005.787 ## ## ## $properties$relativeLocation$properties$bearing ## $properties$relativeLocation$properties$bearing$unitCode ## [1] "wmoUnit:degree_(angle)" ## ## $properties$relativeLocation$properties$bearing$value ## [1] 169 ## ## ## ## ## $properties$forecastZone ## [1] "https://api.weather.gov/zones/forecast/IAZ048" ## ## $properties$county ## [1] "https://api.weather.gov/zones/county/IAC169" ## ## $properties$fireWeatherZone ## [1] "https://api.weather.gov/zones/fire/IAZ048" ## ## $properties$timeZone ## [1] "America/Chicago" ## ## $properties$radarStation ## [1] "KDMX" ``` --- class:inverse ## Your Turn The NOAA Weather API has many layers. If you use the /points/ API and pass in latitude and longitude, `https://api.weather.gov/points/42.0238,-93.6161` the returned JSON file provides links to other APIs. Use the `jsonlite` package to work with the returned JSON file (`jsonlite::fromJSON`). Write a function that accepts latitude and longitude and returns the hourly forecast as a data frame. Can you plot the temperature forecast? --- ## Your Turn - Solution ```r get_weather <- function(lat, lon) { checkmate::assert_number(lat) checkmate::assert_number(lon) url <- sprintf("https://api.weather.gov/points/%f,%f", lat, lon) initialjson <- jsonlite::fromJSON(url) if (!checkmate::check_subset("properties", names(initialjson)) | !checkmate::check_subset("forecastHourly", names(initialjson$properties))) { stop("No Information Found. Check Latitude/Longitude values.") } hourlyforecast <- jsonlite::fromJSON(initialjson$properties$forecastHourly) res <- hourlyforecast$properties$periods checkmate::check_data_frame(res) res } ``` --- ## Your Turn - Solution ```r amesweather <- get_weather(42, -93.6) %>% mutate(startTime = lubridate::ymd_hms(startTime, tz = "America/Chicago")) ``` ``` ## Date in ISO8601 format; converting timezone from UTC to "America/Chicago". ``` 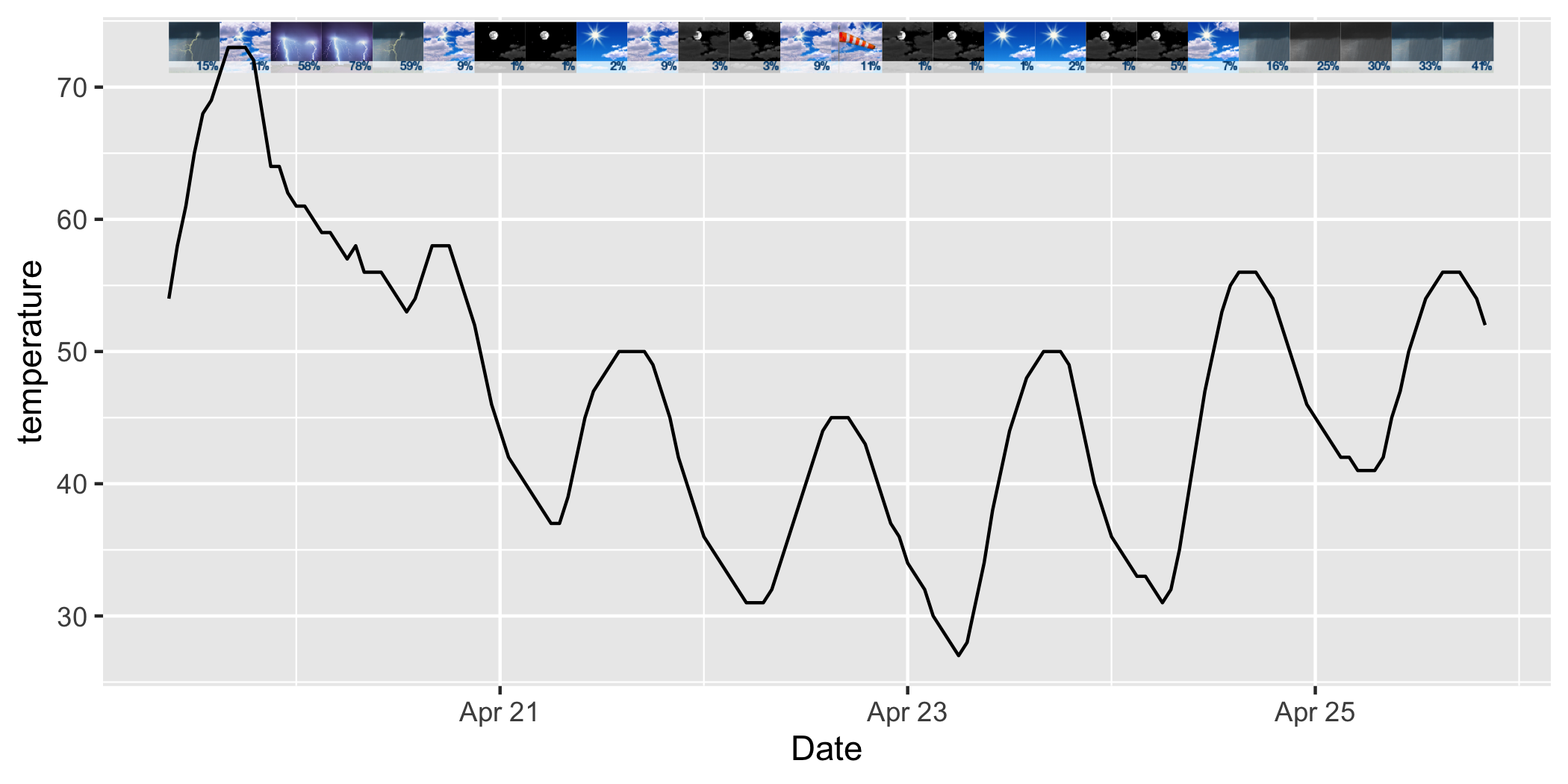<!-- -->